C# winform 自定义messagebox 选项
时间: 2023-07-12 12:08:08 浏览: 296
您可以使用自定义 WinForm 控件来创建自己的 MessageBox,以下是一些实现思路:
1. 创建一个继承自 Form 的类,用于作为自定义 MessageBox 的主界面;
2. 在该界面中添加需要的控件,比如 Label 用于显示提示信息,Button 用于处理用户的选择等;
3. 在调用自定义 MessageBox 的代码中,实例化该 Form 类,并设置其属性和事件处理程序;
4. 在事件处理程序中,获取用户的选择并返回给调用方。
下面是一个简单的示例代码:
```csharp
public partial class CustomMessageBox : Form
{
public CustomMessageBox(string message, string title, MessageBoxButtons buttons)
{
InitializeComponent();
this.lblMessage.Text = message;
this.Text = title;
switch (buttons)
{
case MessageBoxButtons.OK:
this.btnOK.Visible = true;
break;
case MessageBoxButtons.OKCancel:
this.btnOK.Visible = true;
this.btnCancel.Visible = true;
break;
case MessageBoxButtons.YesNo:
this.btnYes.Visible = true;
this.btnNo.Visible = true;
break;
case MessageBoxButtons.YesNoCancel:
this.btnYes.Visible = true;
this.btnNo.Visible = true;
this.btnCancel.Visible = true;
break;
}
}
public DialogResult Result { get; private set; }
private void btnOK_Click(object sender, EventArgs e)
{
this.Result = DialogResult.OK;
this.Close();
}
private void btnCancel_Click(object sender, EventArgs e)
{
this.Result = DialogResult.Cancel;
this.Close();
}
private void btnYes_Click(object sender, EventArgs e)
{
this.Result = DialogResult.Yes;
this.Close();
}
private void btnNo_Click(object sender, EventArgs e)
{
this.Result = DialogResult.No;
this.Close();
}
}
```
调用该自定义 MessageBox 的代码示例如下:
```csharp
var result = new CustomMessageBox("Are you sure?", "Confirm", MessageBoxButtons.YesNo).ShowDialog();
if (result == DialogResult.Yes)
{
// Do something
}
else
{
// Do something else
}
```
阅读全文
相关推荐
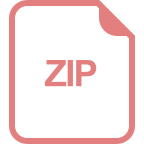
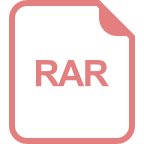


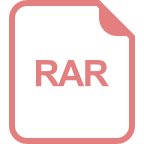
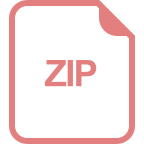
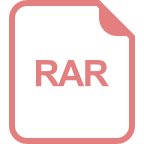
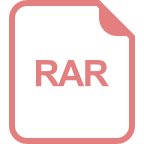
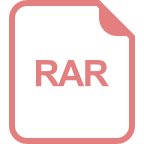
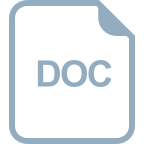
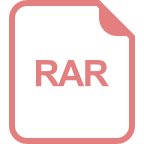
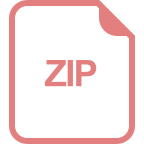
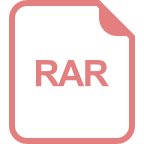
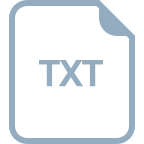
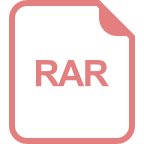
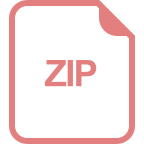
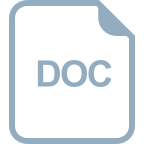
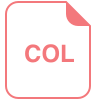