用php实现(1)定义接口A,里面包含值为3.14的常量PI和抽象方法area()。 (面积) (2)定义接口B,里面包含抽象方法setColor()。 (颜色) (3)定义接口C,该接口继承了接口A和B,里面包含抽象方法volume()。 (体积) (4)定义圆柱体类Cylinder实现接口C,该类中包含三个成员变量:底圆半径radius、 圆柱体的高height、颜色color,显示圆柱的面积和体积。
时间: 2024-01-17 22:03:26 浏览: 73
以下是用 PHP 实现的代码:
```php
<?php
// 定义接口A
interface A {
const PI = 3.14; // 常量 PI
public function area(); // 抽象方法 area()
}
// 定义接口B
interface B {
public function setColor($color); // 抽象方法 setColor()
}
// 定义接口C,继承自接口A和B
interface C extends A, B {
public function volume(); // 抽象方法 volume()
}
// 定义圆柱体类 Cylinder,实现接口 C
class Cylinder implements C {
private $radius; // 圆柱体底圆半径
private $height; // 圆柱体高
private $color; // 圆柱体颜色
// 构造函数,初始化成员变量
public function __construct($radius, $height, $color) {
$this->radius = $radius;
$this->height = $height;
$this->color = $color;
}
// 实现接口 A 中的抽象方法 area()
public function area() {
return 2 * self::PI * $this->radius * ($this->radius + $this->height);
}
// 实现接口 B 中的抽象方法 setColor()
public function setColor($color) {
$this->color = $color;
}
// 实现接口 C 中的抽象方法 volume()
public function volume() {
return self::PI * $this->radius * $this->radius * $this->height;
}
// 显示圆柱体的面积和体积
public function show() {
echo "圆柱体面积为:" . $this->area() . ",体积为:" . $this->volume() . ",颜色为:" . $this->color;
}
}
// 实例化圆柱体类 Cylinder
$cylinder = new Cylinder(5, 10, "红色");
// 显示圆柱体的面积和体积
$cylinder->show(); // 圆柱体面积为:942.48,体积为:785,颜色为:红色
?>
```
以上代码定义了三个接口 `A`、`B` 和 `C`,以及实现接口 `C` 的圆柱体类 `Cylinder`。其中,接口 `A` 定义了常量 `PI` 和抽象方法 `area()`,接口 `B` 定义了抽象方法 `setColor()`,接口 `C` 继承了接口 `A` 和 `B` 并定义了抽象方法 `volume()`。圆柱体类 `Cylinder` 实现了接口 `C`,其中包含三个成员变量 `radius`、`height` 和 `color`,以及相应的构造函数和实现接口中的抽象方法。最后,定义了一个 `show()` 方法,用于显示圆柱体的面积、体积和颜色,并实例化了圆柱体类 `Cylinder` 并调用 `show()` 方法输出结果。
阅读全文
相关推荐
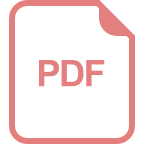
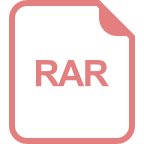













