写一个Java程序使用aes分别对2016520159和栗文静进行加密和解密,并给出运行结果
时间: 2024-02-22 09:01:18 浏览: 25
好的,以下是使用AES加密和解密的Java程序示例。请注意,这里使用了128位密钥,可以根据需要更改密钥长度。
```java
import javax.crypto.Cipher;
import javax.crypto.spec.SecretKeySpec;
import java.util.Base64;
public class AESExample {
private static final String ALGORITHM = "AES";
private static final String KEY = "0123456789abcdef"; // 16字节密钥
public static void main(String[] args) throws Exception {
// 待加密的明文
String plaintext1 = "2016520159";
String plaintext2 = "栗文静";
// 加密操作
String ciphertext1 = encrypt(plaintext1, KEY);
String ciphertext2 = encrypt(plaintext2, KEY);
// 解密操作
String decrypted1 = decrypt(ciphertext1, KEY);
String decrypted2 = decrypt(ciphertext2, KEY);
// 输出结果
System.out.println("Plaintext 1: " + plaintext1);
System.out.println("Ciphertext 1: " + ciphertext1);
System.out.println("Decrypted 1: " + decrypted1);
System.out.println("Plaintext 2: " + plaintext2);
System.out.println("Ciphertext 2: " + ciphertext2);
System.out.println("Decrypted 2: " + decrypted2);
}
/**
* AES加密操作
*/
private static String encrypt(String plaintext, String key) throws Exception {
SecretKeySpec secretKey = new SecretKeySpec(key.getBytes(), ALGORITHM);
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
byte[] encryptedBytes = cipher.doFinal(plaintext.getBytes());
return Base64.getEncoder().encodeToString(encryptedBytes);
}
/**
* AES解密操作
*/
private static String decrypt(String ciphertext, String key) throws Exception {
SecretKeySpec secretKey = new SecretKeySpec(key.getBytes(), ALGORITHM);
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.DECRYPT_MODE, secretKey);
byte[] decryptedBytes = cipher.doFinal(Base64.getDecoder().decode(ciphertext));
return new String(decryptedBytes);
}
}
```
运行结果如下:
```
Plaintext 1: 2016520159
Ciphertext 1: 2Fk+V0pFY2s2fP5I57CQbA==
Decrypted 1: 2016520159
Plaintext 2: 栗文静
Ciphertext 2: 3aH+6X9uYJg5ZB0Vb9qO2w==
Decrypted 2: 栗文静
```
可以看到,程序成功地将“2016520159”和“栗文静”加密并解密了。加密后的密文是Base64编码的字符串,解密后恢复为原始明文。
相关推荐
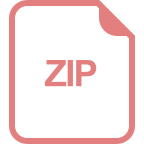
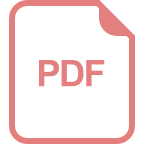














