int * getRandom( ) { static int r[10]; int i; /* 设置种子 */ srand( (unsigned)time( NULL ) ); for ( i = 0; i < 10; ++i) { r[i] = rand(); printf("%d\n", r[i] ); } return r; }
时间: 2024-04-02 13:33:49 浏览: 99
这段代码定义了一个名为 getRandom 的函数,它返回一个 int 指针。该函数内部定义了一个静态整型数组 r,数组长度为 10,用于存储生成的随机数。函数内部使用 srand 函数根据时间设置随机数种子,然后使用 rand 函数生成 10 个随机数,并将这些随机数存储在数组 r 中。最后,函数返回指向数组 r 的指针。由于数组 r 是静态数组,其生命周期与程序运行时间相同,因此可以安全地返回指向数组 r 的指针。在函数外部,可以通过调用 getRandom 函数获取生成的随机数数组。
相关问题
package cloud.cue.group.util; import java.util.Random; /** * @Author xingmengyuan * @Date 2023/5/30 */ public class demo { public static void main(String[] args) { String random = getRandom(7); System.out.println("交换前:"+ random); Integer max = 0; char[] charsA = random.toCharArray(); char[] charsB = random.toCharArray(); for (int i = 0; i < random.length(); i++) { char temp = charsA[i]; if(temp == 0){ continue; } for (int j = i+1; j < random.length(); j++) { if(temp == charsA[j]){ continue; } charsB[i] = charsA[j]; charsB[j] = temp; String tempNum = new String(charsB); Integer newNum = Integer.valueOf(tempNum); if (max.compareTo(newNum) < 0) { max = newNum; } //还原比较对象 charsB = random.toCharArray(); //System.out.println("有效交换次:"+newNum); } } System.out.println("交换后:" + max); } public static String getRandom(int len) { int digit = (int) Math.pow(10, len - 1); int rs = new Random().nextInt(digit * 10); if (rs < digit) { rs += digit; } return String.valueOf(rs); } } 转换成python
import random
def get_random(len):
digit = 10 ** (len - 1)
rs = random.randint(digit, digit * 10 - 1)
if rs < digit:
rs += digit
return str(rs)
def main():
random_str = get_random(7)
print("交换前:" + random_str)
max_num = 0
chars_a = list(random_str)
chars_b = list(random_str)
for i in range(len(random_str)):
temp = chars_a[i]
if temp == '0':
continue
for j in range(i + 1, len(random_str)):
if temp == chars_a[j]:
continue
chars_b[i] = chars_a[j]
chars_b[j] = temp
temp_num = ''.join(chars_b)
new_num = int(temp_num)
if max_num < new_num:
max_num = new_num
# 还原比较对象
chars_b = list(random_str)
# print("有效交换次:" + str(new_num))
print("交换后:" + str(max_num))
if __name__ == '__main__':
main()
分析以下代码:class RandomizedSet { public: RandomizedSet() { vector<int> *c=new vector<int>; } bool insert(int val) { c.push_bakc(val); return true; } bool remove(int val) { auto n=c.find(val); if(n==c.end()) return false; c.erase(n); return true; } int getRandom() { return c[random()%c.size()]; } };
这是一个实现了随机集合(RandomizedSet)功能的类,其中包含了以下成员函数:
- 构造函数: RandomizedSet(),用于初始化一个vector<int>类型的指针成员变量c。
- insert函数: bool insert(int val),用于向随机集合中插入一个元素val,将val插入到vector<int>类型的指针成员变量c的末尾,并返回true。
- remove函数: bool remove(int val),用于从随机集合中移除一个元素val,查找val在vector<int>类型的指针成员变量c中的位置,如果未找到则返回false,否则从c中移除该元素并返回true。
- getRandom函数: int getRandom(),用于随机获取集合中的一个元素,返回vector<int>类型的指针成员变量c中随机位置的元素。
上述代码中存在以下问题:
1. 在构造函数中使用了new操作符动态分配内存,但没有对其进行释放,会导致内存泄漏。
2. insert函数中使用了c.push_bakc(val),但c的类型为vector<int>*,应该使用箭头运算符->来访问成员函数。
3. getRandom函数中使用了random()函数,但该函数没有定义,应该使用std::rand()函数或者C++11中的std::mt19937随机数生成器。
阅读全文
相关推荐
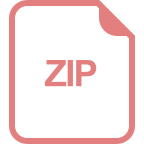
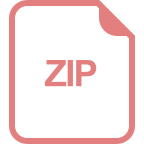
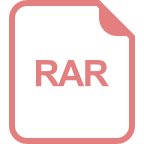
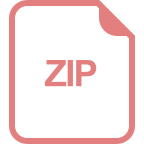









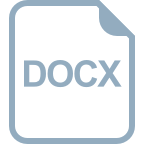