怎么使用jquery发送ajax请求
时间: 2023-09-22 18:07:04 浏览: 61
使用jQuery发送Ajax请求有以下几个步骤:
1. 引入jQuery库文件。在HTML文件中添加以下代码:
```html
<script src="https://cdn.jsdelivr.net/jquery/3.5.1/jquery.min.js"></script>
```
2. 编写Ajax请求。可以使用`$.ajax()`或`$.get()`、`$.post()`等方法。
`$.ajax()`方法的基本语法:
```javascript
$.ajax({
url: 请求地址,
type: 请求方式,
data: 请求参数,
dataType: 返回数据类型,
success: 成功回调函数,
error: 失败回调函数
})
```
示例:
```javascript
$.ajax({
url: 'http://example.com/api',
type: 'POST',
data: {param1: 'value1', param2: 'value2'},
dataType: 'json',
success: function(response) {
console.log(response);
},
error: function(xhr, status, error) {
console.log('请求失败:' + error);
}
});
```
`$.get()`和`$.post()`方法的基本语法:
```javascript
$.get(url, data, success, dataType);
$.post(url, data, success, dataType);
```
示例:
```javascript
$.get('http://example.com/api', {param1: 'value1', param2: 'value2'}, function(response) {
console.log(response);
}, 'json');
$.post('http://example.com/api', {param1: 'value1', param2: 'value2'}, function(response) {
console.log(response);
}, 'json');
```
其中,`url`为请求的地址,`type`或方法名为请求的方式(GET或POST),`data`为请求的参数,`dataType`为期望的返回数据类型(可选,默认为智能判断),`success`为请求成功后的回调函数,`error`为请求失败后的回调函数。
相关推荐
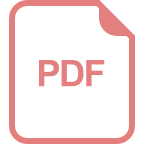














