springboot vue 图片上传功能
时间: 2023-06-17 14:06:39 浏览: 134
实现 Spring Boot 后端和 Vue.js 前端的图片上传功能可以分为以下几个步骤:
1. 创建 Spring Boot 后端接收图片的 API。可以使用 Spring Boot 的 `@PostMapping` 注解和 `@RequestParam` 注解来接收上传的图片文件,然后将文件保存到服务器的本地磁盘或者云存储中,返回保存成功的图片路径。
示例代码:
```java
@PostMapping("/uploadImage")
public String uploadImage(@RequestParam("file") MultipartFile file) throws IOException {
// 保存文件
String fileName = file.getOriginalFilename();
String filePath = "/path/to/image/directory/" + fileName;
File dest = new File(filePath);
file.transferTo(dest);
// 返回保存成功的图片路径
return filePath;
}
```
2. 创建 Vue.js 前端上传图片的组件,并在组件中使用 `axios` 库发送请求到后端接口上传图片文件。
示例代码:
```vue
<template>
<div>
<input type="file" ref="fileInput" @change="handleFileChange">
<button @click="uploadImage">上传图片</button>
</div>
</template>
<script>
import axios from 'axios';
export default {
data() {
return {
file: null,
imageUrl: ''
}
},
methods: {
handleFileChange(event) {
this.file = event.target.files[0];
},
uploadImage() {
let formData = new FormData();
formData.append('file', this.file);
axios.post('/api/uploadImage', formData, {
headers: {
'Content-Type': 'multipart/form-data'
}
}).then(response => {
this.imageUrl = response.data;
console.log('Image uploaded successfully: ' + this.imageUrl);
}).catch(error => {
console.log('Error uploading image: ' + error);
});
}
}
}
</script>
```
其中 `handleFileChange` 方法会在用户选择要上传的图片文件时被触发,将选中的文件保存到组件的 `file` 属性中。`uploadImage` 方法会创建一个 `FormData` 对象,将组件的 `file` 属性添加到其中,然后使用 `axios` 发送 POST 请求到后端接口上传图片文件,并将上传成功后返回的图片路径保存到组件的 `imageUrl` 属性中。
3. 在 Vue.js 前端页面中显示上传成功的图片。
示例代码:
```vue
<template>
<div>
<input type="file" ref="fileInput" @change="handleFileChange">
<button @click="uploadImage">上传图片</button>
<img :src="imageUrl">
</div>
</template>
<script>
import axios from 'axios';
export default {
data() {
return {
file: null,
imageUrl: ''
}
},
methods: {
handleFileChange(event) {
this.file = event.target.files[0];
},
uploadImage() {
let formData = new FormData();
formData.append('file', this.file);
axios.post('/api/uploadImage', formData, {
headers: {
'Content-Type': 'multipart/form-data'
}
}).then(response => {
this.imageUrl = response.data;
console.log('Image uploaded successfully: ' + this.imageUrl);
}).catch(error => {
console.log('Error uploading image: ' + error);
});
}
}
}
</script>
```
其中 `<img :src="imageUrl">` 标签用来显示上传成功的图片,其 `src` 属性绑定到组件的 `imageUrl` 属性,当 `imageUrl` 属性更新时,图片会自动刷新。
阅读全文
相关推荐





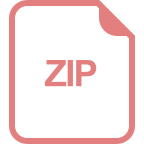
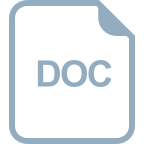





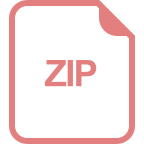
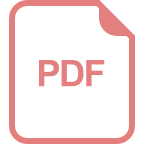
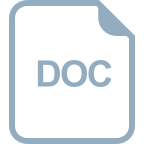
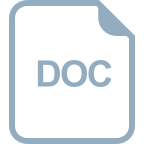