一个带权无向图,地点名字已经用一维数组存储了,距离已经用二维数组存储了,用Java实现它的最短路径查询
时间: 2024-12-22 11:31:23 浏览: 0
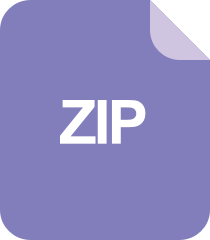
无向图pagerank算法(Java)
在Java中,我们可以使用Dijkstra算法来解决这个问题,它是最有效的求解带权重无向图中最短路径的问题之一。首先,我们需要创建一个`Node`类表示图中的每个节点,包含名字和到达其他节点的距离信息。然后,使用一个优先队列(如`PriorityQueue`或自定义的`Heap`)来组织并更新最近发现的节点。
以下是一个简单的步骤概述:
1. 定义`Node`类:
```java
class Node {
String name;
int distance; // 初始距离设为无穷大
List<Node> neighbors; // 存储邻居及它们之间的距离
public Node(String name) {
this.name = name;
distance = Integer.MAX_VALUE;
}
}
```
2. 创建一个`Graph`类,使用邻接表存储图结构,并实现Dijkstra算法:
```java
import java.util.*;
class Graph {
private Map<String, Node> nodes;
public Graph(String[] locations, int[][] distances) {
nodes = new HashMap<>();
for (int i = 0; i < locations.length; i++) {
nodes.put(locations[i], new Node(locations[i]));
}
populateNeighbors(distances);
}
private void populateNeighbors(int[][] distances) {
for (int i = 0; i < distances.length; i++) {
for (int j = i + 1; j < distances[i].length; j++) {
if (distances[i][j] != 0) { // 非零距离才添加边
nodes.get(locations[i]).neighbors.add(nodes.get(locations[j]), distances[i][j]);
nodes.get(locations[j]).neighbors.add(nodes.get(locations[i]), distances[i][j]); // 对称性,无向图
}
}
}
}
public List<Node> dijkstra(String startNode) {
// 更详细的实现会包括优先队列操作、松弛操作等
// ...
}
}
// 示例:
public class Main {
public static void main(String[] args) {
String[] locations = {"A", "B", "C", "D"};
int[][] distances = {{0, 5, 7, 9},
{5, 0, 10, 8},
{7, 10, 0, 6},
{9, 8, 6, 0}};
Graph graph = new Graph(locations, distances);
List<Node> shortestPath = graph.dijkstra("A");
// 最短路径在这里...
}
}
```
在这个示例中,`dijkstra`方法将返回从指定起点到所有其他节点的最短路径列表。
阅读全文
相关推荐
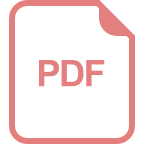
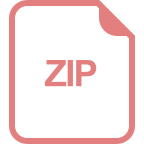
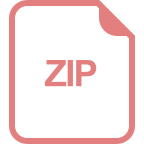
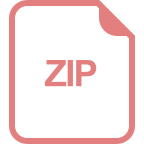
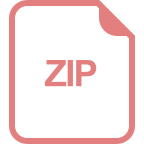
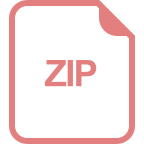
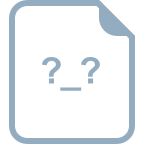