在eclipse中怎么用mysql语句实现增填
时间: 2024-05-22 20:14:05 浏览: 44
您可以使用 JDBC 驱动程序和 MySQL 连接,以便在 Eclipse 中使用 MySQL 语句实现增添数据。以下是一些基本步骤:
1. 下载和设置 MySQL JDBC 驱动程序。
2. 创建一个连接对象来连接到 MySQL 数据库。
3. 编写 SQL 语句来执行插入操作。
4. 使用 PreparedStatement 或 Statement 对象来执行 SQL 语句。
5. 关闭连接对象和执行对象以保持数据的完整性。
希望以上内容能对您有所帮助。
相关问题
eclipse中的mysql查询语句
Eclipse中使用Maven或直接配置JDBC连接MySQL数据库,你可以编写SQL查询语句来操作数据。以下是基本步骤:
1. **添加MySQL驱动**:确保Eclipse的项目中已经添加了MySQL JDBC驱动,可以在pom.xml文件(如果使用Maven)或src/main/resources/META-INF下手动添加mysql-connector-java.jar。
2. **配置连接信息**:创建`DataSource`对象,需要提供URL、用户名、密码等信息。例如:
```java
String url = "jdbc:mysql://localhost:3306/mydatabase";
String user = "root";
String password = "mypassword";
Connection connection = DriverManager.getConnection(url, user, password);
```
3. **编写查询语句**:
- 使用PreparedStatement防止SQL注入,并能获取预编译的语句性能优势:
```java
String query = "SELECT * FROM mytable WHERE id = ?";
PreparedStatement pstmt = connection.prepareStatement(query);
pstmt.setInt(1, someId);
ResultSet resultSet = pstmt.executeQuery();
```
- 或者使用Statement执行简单的查询:
```java
String query = "SELECT * FROM mytable WHERE name = 'John'";
Statement stmt = connection.createStatement();
ResultSet resultSet = stmt.executeQuery(query);
```
4. **处理结果集**:
遍历ResultSet,获取你需要的数据。
怎么在eclipse中获取mysql中的信息并输出到页面上
你可以使用 Java 中的 JDBC API 连接 MySQL 数据库,并执行 SQL 查询语句,然后将查询结果输出到页面上。
首先,你需要在 Eclipse 中添加 MySQL 的 JDBC 驱动程序。可以下载 MySQL Connector/J 驱动程序并将其添加到项目的类路径中。然后,你需要编写 Java 代码来连接 MySQL 数据库,执行查询语句并处理结果集。
以下是一个示例代码,可以查询一个表中的数据并将其输出到控制台:
```
import java.sql.*;
public class MySQLTest {
public static void main(String[] args) {
try {
// 连接 MySQL 数据库
Class.forName("com.mysql.jdbc.Driver");
Connection conn = DriverManager.getConnection(
"jdbc:mysql://localhost:3306/mydatabase", "username", "password");
// 执行 SQL 查询语句
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery("SELECT * FROM mytable");
// 处理查询结果集
while (rs.next()) {
int id = rs.getInt("id");
String name = rs.getString("name");
int age = rs.getInt("age");
System.out.println("ID: " + id + ", Name: " + name + ", Age: " + age);
}
// 关闭连接
rs.close();
stmt.close();
conn.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
你可以根据需要修改代码,并将查询结果输出到网页上。例如,你可以使用 JSP 或 Servlet 技术来实现页面输出。
阅读全文
相关推荐
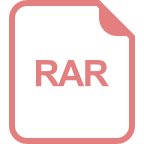
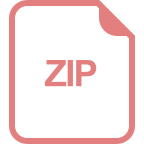
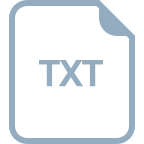
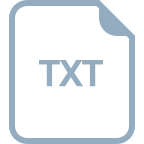
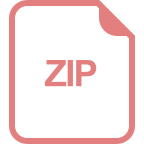
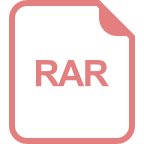
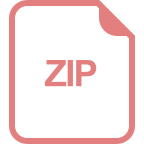
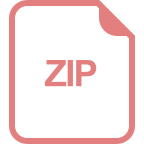
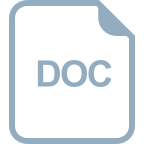
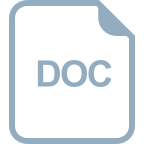




