with open(filename, 'r', encoding='UTF-8') as fp: 为什么要用 encoding='UTF-8'
时间: 2024-01-24 13:05:40 浏览: 103
在 Python 2.x 版本中,默认的编码方式是 ASCII,但在 Python 3.x 版本中,默认的编码方式是 UTF-8。因此,如果我们在 Python 3.x 版本中打开一个文件时,不指定编码方式,那么默认的编码方式会是 UTF-8。但是,为了增强代码的可读性,我们一般会在打开文件时显式地指定编码方式,以确保读取文件时使用正确的编码方式。因此,我们使用 `encoding='UTF-8'` 参数来告诉 Python 解释器,我们要以 UTF-8 编码方式打开这个文件。
相关问题
用python完成实践(中文词频统计):对中文词频统计项目(import jieba import wordcloud class ChineseWordCounter: def __init__(self): self.content = '' self.words = [] self.word_frequencies = {} def readfile_demo_with(self, filename): with open(filename, encoding='utf-8') as fp: self.content = fp.read() def seperate_words(self): ignore_word = ('的', '与', '个', '和') for word in jieba.cut(self.content, cut_all=False): if (len(word) > 1) and (word not in ignore_word): self.words.append(word) def count_words(self): for word in self.words: if word in self.word_frequencies.keys(): self.word_frequencies[word] += 1 else: self.word_frequencies[word] = 1 def draw_wordcloud(self, img_file): font = 'c:/windows/font/方正粗黑宋简体.ttf' cloudengine = wordcloud.WordCloud(font_path=font) cloudengine.generate(" ".join(self.words)) cloudengine.to_file(img_file))进行修改,把统计出的词频保存到指定的csv文件中。
你可以按照以下方式修改代码,将统计出的词频保存到指定的CSV文件中:
```python
import csv
import jieba
import wordcloud
class ChineseWordCounter:
def __init__(self):
self.content = ''
self.words = []
self.word_frequencies = {}
def readfile_demo_with(self, filename):
with open(filename, encoding='utf-8') as fp:
self.content = fp.read()
def separate_words(self):
ignore_word = ('的', '与', '个', '和')
for word in jieba.cut(self.content, cut_all=False):
if (len(word) > 1) and (word not in ignore_word):
self.words.append(word)
def count_words(self):
for word in self.words:
if word in self.word_frequencies.keys():
self.word_frequencies[word] += 1
else:
self.word_frequencies[word] = 1
def save_word_frequencies_to_csv(self, csv_file):
with open(csv_file, mode='w', encoding='utf-8', newline='') as file:
writer = csv.writer(file)
writer.writerow(['Word', 'Frequency'])
for word, freq in self.word_frequencies.items():
writer.writerow([word, freq])
def draw_wordcloud(self, img_file):
font = 'c:/windows/font/方正粗黑宋简体.ttf'
cloudengine = wordcloud.WordCloud(font_path=font)
cloudengine.generate(" ".join(self.words))
cloudengine.to_file(img_file)
```
你可以调用 `save_word_frequencies_to_csv` 方法,将词频保存到指定的 CSV 文件中。例如,假设你的实例名为 `counter`,你可以通过 `counter.save_word_frequencies_to_csv('word_frequencies.csv')` 来保存词频到 `word_frequencies.csv` 文件中。
解释这行代码from os import listdir from os.path import isdir, join notRepeatedLines = set() # 保存非重复的代码行 file_num, code_num = 0, 0 # 文件数量与代码总行数 def linesCount(directory): global file_num, code_num for filename in listdir(directory): temp = join(directory, filename) if isdir(temp): # 递归遍历子文件夹 linesCount(temp) elif temp.endswith('.cpp'): # 只考虑.cpp文件 file_num += 1 with open(temp, 'r', encoding='utf8') as fp: for line in fp: notRepeatedLines.add(line.strip()) code_num += 1 # 记录所有代码行 linesCount('F:\教学课件\计算机图形学') print('总行数:{0},非重复行数:{1}'.format(code_num, len(notRepeatedLines))) print('文件数量:{0}'.format(file_num))
这段代码的主要功能是统计某个文件夹下所有.cpp文件的代码行数和非重复行数。
1. `from os import listdir from os.path import isdir, join`
这行代码导入了os模块中的listdir函数和os.path模块中的isdir和join函数。
2. `notRepeatedLines = set()`
定义了一个集合用于保存非重复的代码行。
3. `file_num, code_num = 0, 0`
初始化文件数量和代码总行数为0。
4. `def linesCount(directory):`
定义了一个函数linesCount,用于递归遍历指定目录下的所有文件和子文件夹,并统计代码行数和非重复行数。
5. `global file_num, code_num`
在函数内部声明变量file_num和code_num为全局变量,以便在函数内部修改这些变量的值。
6. `for filename in listdir(directory):`
遍历指定目录下的所有文件和文件夹。
7. `temp = join(directory, filename)`
使用os.path.join函数拼接文件路径。
8. `if isdir(temp):`
如果当前文件是一个文件夹,则递归调用linesCount函数。
9. `elif temp.endswith('.cpp'):`
如果当前文件是一个.cpp文件,则进行代码行统计。
10. `file_num += 1`
文件数量加1。
11. `with open(temp, 'r', encoding='utf8') as fp:`
打开当前文件,并使用with语句自动关闭文件。
12. `for line in fp:`
遍历文件中的每一行。
13. `notRepeatedLines.add(line.strip())`
将当前行去除首尾空格后添加到非重复行集合中。
14. `code_num += 1`
代码总行数加1。
15. `linesCount('F:\教学课件\计算机图形学')`
调用函数linesCount,开始遍历指定目录下的所有文件和子文件夹。
16. `print('总行数:{0},非重复行数:{1}'.format(code_num, len(notRepeatedLines)))`
输出代码总行数和非重复行数。
17. `print('文件数量:{0}'.format(file_num))`
输出文件数量。
阅读全文
相关推荐
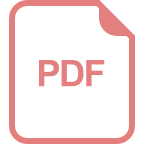
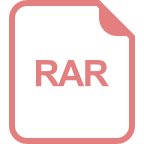



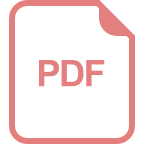
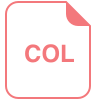
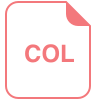
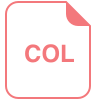
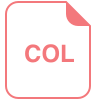
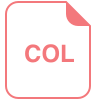
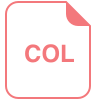
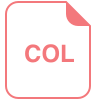
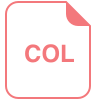

