使用QT实现存放与服务器端的音视频文件预览功能
时间: 2023-05-28 13:07:21 浏览: 144
要实现存放与服务器端的音视频文件预览功能,可以使用Qt框架中的多媒体模块和网络模块。
首先,需要使用Qt的网络模块连接到服务器端,获取音视频文件的URL或文件路径。然后,使用Qt的多媒体模块创建一个音视频播放器,并将URL或文件路径传递给该播放器。最后,在Qt的GUI界面中添加一个视频窗口,将音视频播放器的输出与该窗口关联起来,即可实现音视频文件的预览功能。
以下是一个简单的示例代码,演示了如何使用Qt实现存放与服务器端的音视频文件预览功能:
```cpp
#include <QtWidgets>
#include <QtNetwork>
#include <QtMultimedia>
class VideoPlayer : public QWidget
{
public:
VideoPlayer(QWidget *parent = nullptr)
: QWidget(parent)
{
// 创建多媒体播放器
m_player = new QMediaPlayer(this);
// 创建视频窗口
m_videoWidget = new QVideoWidget(this);
m_player->setVideoOutput(m_videoWidget);
// 创建布局
QVBoxLayout *layout = new QVBoxLayout;
layout->addWidget(m_videoWidget);
setLayout(layout);
}
void play(const QUrl &url)
{
// 设置媒体源
m_player->setMedia(url);
// 播放媒体
m_player->play();
}
private:
QMediaPlayer *m_player;
QVideoWidget *m_videoWidget;
};
class FilePreviewer : public QWidget
{
public:
FilePreviewer(QWidget *parent = nullptr)
: QWidget(parent)
{
// 创建GUI界面
m_label = new QLabel(tr("Enter file URL:"));
m_lineEdit = new QLineEdit;
m_button = new QPushButton(tr("Play"));
m_videoPlayer = new VideoPlayer;
QHBoxLayout *layout = new QHBoxLayout;
layout->addWidget(m_label);
layout->addWidget(m_lineEdit);
layout->addWidget(m_button);
QVBoxLayout *mainLayout = new QVBoxLayout;
mainLayout->addLayout(layout);
mainLayout->addWidget(m_videoPlayer);
setLayout(mainLayout);
// 连接信号和槽
connect(m_button, &QPushButton::clicked, this, &FilePreviewer::play);
}
private:
QLabel *m_label;
QLineEdit *m_lineEdit;
QPushButton *m_button;
VideoPlayer *m_videoPlayer;
void play()
{
// 获取文件URL
QUrl url = m_lineEdit->text();
// 播放文件
m_videoPlayer->play(url);
}
};
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
FilePreviewer previewer;
previewer.show();
return app.exec();
}
```
在上述代码中,`VideoPlayer`类负责创建多媒体播放器和视频窗口,并将它们关联起来。`FilePreviewer`类负责创建GUI界面和处理用户输入,当用户点击“播放”按钮时,它会获取文件URL并将其传递给`VideoPlayer`类的实例进行播放。在`main()`函数中,我们创建了一个`FilePreviewer`类的实例,并将其显示在屏幕上。
需要注意的是,以上示例代码仅供参考,实际应用中需要根据具体需求进行适当修改。例如,如果要支持存放在服务器端的音视频文件,需要使用Qt的网络模块连接到服务器端并获取文件URL。另外,还需要处理网络连接的错误和超时等异常情况。
相关推荐
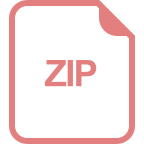














