设计一个算法在广义表中查找一个特定值的元素是否存在(用C语言编写)
时间: 2024-10-19 15:11:32 浏览: 44
设计一个在广义表(也称作链表、树形结构等)中查找特定值的算法,通常我们可以选择递归的方式来遍历整个列表。这里是一个简单的C语言版本示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义广义表节点结构体
typedef struct ListNode {
int value; // 节点值
struct ListNode* next; // 指向下一个节点的指针
} ListNode;
// 函数声明
ListNode* searchInList(ListNode* head, int target); // 查找函数
// 用于创建新节点
ListNode* createNode(int value) {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
newNode->value = value;
newNode->next = NULL;
return newNode;
}
// 主函数 - 查找并打印结果
void findElement(ListNode* list, int target) {
ListNode* result = searchInList(list, target);
if (result != NULL) {
printf("找到元素 %d\n", target);
} else {
printf("元素 %d 未找到\n", target);
}
}
// 递归查找函数
ListNode* searchInList(ListNode* head, int target) {
if (head == NULL || head->value == target) { // 如果到达末尾或找到目标值,返回对应节点
return head;
}
// 递归搜索下一个节点
ListNode* foundNode = searchInList(head->next, target);
return foundNode; // 返回最终找到的节点
}
int main() {
// 创建一个测试广义表
ListNode* testList = createNode(10);
testList->next = createNode(20);
testList->next->next = createNode(30);
testList->next->next->next = createNode(40);
// 找元素示例
findElement(testList, 20);
return 0;
}
```
在这个示例中,`searchInList`函数会递归地遍历列表,直到找到目标值或者遍历完整个列表。如果没有找到,它将返回NULL。
阅读全文
相关推荐






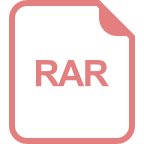


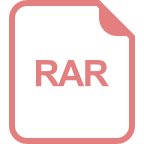
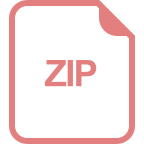
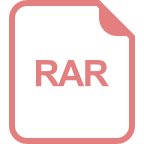





