Java 比较两个list中元素的属性是否相同
时间: 2023-08-06 14:03:28 浏览: 53
在Java中,比较两个list中元素的属性是否相同,可以使用以下代码:
```
public static <T> boolean compareLists(List<T> list1, List<T> list2, String property) throws NoSuchFieldException, IllegalAccessException {
if (list1.size() != list2.size()) {
return false;
}
for (int i = 0; i < list1.size(); i++) {
T obj1 = list1.get(i);
T obj2 = list2.get(i);
Field field = obj1.getClass().getDeclaredField(property);
field.setAccessible(true);
Object value1 = field.get(obj1);
Object value2 = field.get(obj2);
if (!Objects.equals(value1, value2)) {
return false;
}
}
return true;
}
// 示例代码
public static void main(String[] args) throws NoSuchFieldException, IllegalAccessException {
List<Fruit> list1 = new ArrayList<>();
list1.add(new Fruit("apple", 1.5));
list1.add(new Fruit("banana", 2.0));
list1.add(new Fruit("orange", 1.2));
List<Fruit> list2 = new ArrayList<>();
list2.add(new Fruit("apple", 1.5));
list2.add(new Fruit("banana", 2.0));
list2.add(new Fruit("orange", 1.2));
boolean result1 = compareLists(list1, list2, "name");
System.out.println(result1); // true
List<Fruit> list3 = new ArrayList<>();
list3.add(new Fruit("apple", 1.5));
list3.add(new Fruit("kiwi", 1.8));
list3.add(new Fruit("orange", 1.2));
boolean result2 = compareLists(list1, list3, "name");
System.out.println(result2); // false
}
// 示例类
public class Fruit {
private String name;
private double weight;
public Fruit(String name, double weight) {
this.name = name;
this.weight = weight;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getWeight() {
return weight;
}
public void setWeight(double weight) {
this.weight = weight;
}
}
```
这段代码会先判断两个list的长度是否相等,如果不相等,则直接返回false。如果长度相等,则遍历这两个list中的所有元素,根据传入的property属性来比较它们是否相等。在Java中,我们需要使用反射来获取对象的属性值,因此代码稍微有些复杂。如果两个属性值相等,则返回true,否则返回false。
相关推荐
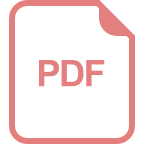
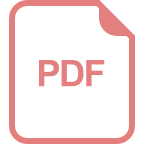














