用vue写一个前端分页
时间: 2024-01-05 22:02:39 浏览: 21
好的,这里提供一个简单的Vue前端分页示例:
1. 在Vue组件中定义数据和方法
```
<template>
<div>
<table>
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Email</th>
<th>Phone</th>
</tr>
</thead>
<tbody>
<tr v-for="(item, index) in paginatedData" :key="index">
<td>{{ item.id }}</td>
<td>{{ item.name }}</td>
<td>{{ item.email }}</td>
<td>{{ item.phone }}</td>
</tr>
</tbody>
</table>
<div class="pagination">
<ul>
<li v-for="pageNumber in totalPages" :key="pageNumber" @click="changePage(pageNumber)" :class="{active: currentPage === pageNumber}">{{ pageNumber }}</li>
</ul>
</div>
</div>
</template>
<script>
export default {
data() {
return {
data: [], // 所有数据
pageSize: 10, // 每页显示的数据量
currentPage: 1, // 当前页码
totalData: 0 // 总数据量
}
},
computed: {
// 计算总页数
totalPages() {
return Math.ceil(this.totalData / this.pageSize);
},
// 计算当前页显示的数据
paginatedData() {
const start = (this.currentPage - 1) * this.pageSize;
const end = start + this.pageSize;
return this.data.slice(start, end);
}
},
methods: {
// 切换页码
changePage(pageNumber) {
this.currentPage = pageNumber;
}
}
}
</script>
```
2. 在父组件中传递数据
```
<template>
<div>
<child-component :data="data"></child-component>
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
data() {
return {
data: [] // 所有数据
}
},
components: {
'child-component': ChildComponent
},
mounted() {
// 获取数据
this.getData();
},
methods: {
// 获取数据
getData() {
// 发送Ajax请求,获取数据
// ...
// 将获取到的数据赋值给this.data
this.data = responseData;
}
}
}
</script>
```
这样,就可以在前端实现一个简单的分页功能了。
阅读全文
相关推荐
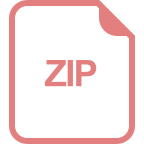
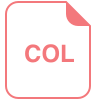



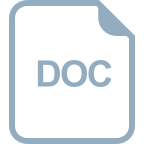
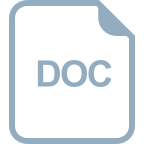







