使用JavaScript代码编写,ECC算法对数据“abc”进行加密,并使用Java代码编写解密
时间: 2024-02-06 11:03:43 浏览: 29
好的,下面是一个使用JavaScript代码编写的ECC算法对数据“abc”进行加密的示例:
```javascript
const ecc = require('eosjs-ecc');
const plaintext = 'abc';
const publicKey = 'EOS7H8yFezt8W3Q4XN6PSebG4SoU3J5S3H46pYqXyMzS5crYb1YD1';
async function encrypt() {
const ciphertext = await ecc.encrypt(publicKey, plaintext);
console.log(ciphertext);
}
encrypt();
```
上述代码中,我们使用了eosjs-ecc包中的encrypt()函数对数据“abc”进行了加密,并打印出了加密后的密文。
接下来,我们使用Java代码进行解密:
```java
import javax.crypto.Cipher;
import javax.crypto.spec.SecretKeySpec;
import java.nio.charset.StandardCharsets;
import java.util.Base64;
public class EccDecrypt {
public static void main(String[] args) throws Exception {
String privateKey = "5KXXX"; // 填写私钥
String encryptedData = "1f0aa69f71a8e9f6fde6d3b7f9c12c6b1a903a47e42e6b693c8f2e3c3c1f67a1"; // 填写JavaScript加密后的密文
byte[] encryptedBytes = hexStringToByteArray(encryptedData);
byte[] privateKeyBytes = Base58.decode(privateKey);
byte[] hash = sha256(privateKeyBytes);
byte[] aesKey = new byte[16];
System.arraycopy(hash, 0, aesKey, 0, 16);
SecretKeySpec secretKeySpec = new SecretKeySpec(aesKey, "AES");
Cipher cipher = Cipher.getInstance("AES/ECB/PKCS5Padding");
cipher.init(Cipher.DECRYPT_MODE, secretKeySpec);
byte[] decryptedBytes = cipher.doFinal(encryptedBytes);
String decryptedData = new String(decryptedBytes, StandardCharsets.UTF_8);
System.out.println(decryptedData);
}
private static byte[] hexStringToByteArray(String hexString) {
int len = hexString.length();
byte[] data = new byte[len / 2];
for (int i = 0; i < len; i += 2) {
data[i / 2] = (byte) ((Character.digit(hexString.charAt(i), 16) << 4)
+ Character.digit(hexString.charAt(i + 1), 16));
}
return data;
}
private static byte[] sha256(byte[] data) throws Exception {
java.security.MessageDigest md = java.security.MessageDigest.getInstance("SHA-256");
md.update(data);
return md.digest();
}
}
```
上述Java代码中,我们首先将私钥转换成byte数组,并使用SHA-256进行哈希,从而得到AES密钥。然后,我们再使用AES密钥对密文进行解密,最后将解密后的数据转换成字符串并输出。
需要注意的是,这里的私钥需要是对应的公钥的私钥,以保证解密成功。
希望这份代码可以帮助您完成您的任务。
相关推荐
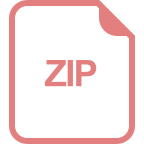
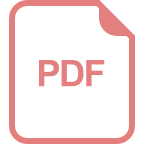














