使用c语言实现,用结构体类型编写一个程序,输入n个客户的姓名和电话,然后按姓名的字典顺序排列后顺序输出
时间: 2024-10-08 18:23:34 浏览: 22
在C语言中,你可以使用结构体(struct)来创建一个客户记录的数据类型,结合数组或动态内存分配来存储数据,并利用qsort函数来进行字典序排序。下面是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 客户结构体
typedef struct {
char name[50]; // 姓名
char phone[15]; // 电话号码
} Customer;
// 比较函数,用于qsort排序
int compare(const void *a, const void *b) {
const Customer *customerA = (const Customer *)a;
const Customer *customerB = (const Customer *)b;
return strcmp(customerA->name, customerB->name); // 字符串比较
}
int main() {
int n;
printf("请输入客户数量: ");
scanf("%d", &n);
// 动态分配内存
Customer *customers = malloc(n * sizeof(Customer));
if (!customers) {
perror("Memory allocation failed");
return 1;
}
for (int i = 0; i < n; i++) {
printf("请输入第%d位客户的姓名: ", i + 1);
fgets(customers[i].name, sizeof(customers[i].name), stdin);
customers[i].name[strcspn(customers[i].name, "\n")] = '\0'; // 移除换行符
printf("请输入第%d位客户的电话: ", i + 1);
fgets(customers[i].phone, sizeof(customers[i].phone), stdin);
customers[i].phone[strcspn(customers[i].phone, "\n")] = '\0'; // 移除换行符
}
// 排序
qsort(customers, n, sizeof(Customer), compare);
// 输出排序后的结果
printf("\n按照姓名字典顺序排列后的客户信息:\n");
for (int i = 0; i < n; i++) {
printf("姓名: %s, 电话: %s\n", customers[i].name, customers[i].phone);
}
// 释放内存
free(customers);
return 0;
}
相关推荐
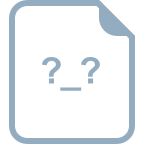
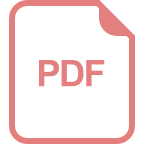
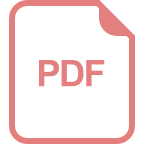














