如何用C语言编写一个算法,基于给定的单链表数据结构,首先创建该链表,然后删除所有值在emin和emax范围之外的节点?请提供必要的数据结构定义和相应的代码实现步骤。
时间: 2024-12-17 14:54:49 浏览: 18
在C语言中,我们可以使用结构体来表示链表节点,并定义两个指针变量作为头结点。这里是一个基本的步骤来实现这个功能:
1. 定义链表节点结构体(Node):
```c
typedef struct Node {
int data;
struct Node* next;
} Node;
```
2. 创建链表函数(createList):
```c
Node* createList(int arr[], int n, int emin, int emax) {
Node* head = NULL;
for (int i = 0; i < n; ++i) {
if (arr[i] >= emin && arr[i] <= emax) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = arr[i];
newNode->next = head;
head = newNode;
}
}
return head;
}
```
3. 删除超出范围节点的函数(deleteOutliers):
```c
void deleteOutliers(Node* head, int emin, int emax) {
if (head == NULL) return;
Node* currentNode = head;
while (currentNode != NULL) {
Node* temp = currentNode;
if (currentNode->data < emin || currentNode->data > emax) {
// 将当前节点的下一个节点赋值给当前节点,然后释放当前节点
currentNode = currentNode->next;
free(temp);
} else {
currentNode = currentNode->next;
}
}
}
```
4. 使用链表(示例):
```c
int main() {
int arr[] = {1, 5, 8, 2, 9, 3, 7, 6, 4};
int n = sizeof(arr) / sizeof(arr[0]);
int emin = 2, emax = 7;
Node* list = createList(arr, n, emin, emax);
deleteOutliers(list, emin, emax);
// 打印修改后的链表(这里仅用于演示,实际应用中无需打印)
Node* temp = list;
while (temp != NULL) {
printf("%d ", temp->data);
temp = temp->next;
}
return 0;
}
```
在这个例子中,`createList` 函数会根据给定数组创建链表,只包含emin和emax范围内的元素;`deleteOutliers` 函数则遍历链表并删除超出范围的节点。
阅读全文
相关推荐
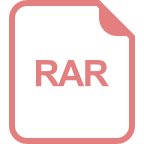
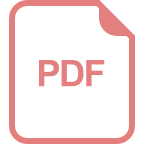
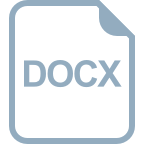















