img 使用post 请求图片
时间: 2024-09-22 09:00:50 浏览: 57
在Web开发中,`img` 标签通常用于显示图像,它本身并不支持发送POST请求加载图片。如果你想通过POST请求获取图片并展示,一般会在服务器端处理好图片数据,然后提供一个URL供前端访问。
前端如果需要动态上传或处理服务器返回的图片,可以采用其他方式进行交互,比如使用JavaScript的fetch API、jQuery的`$.get()`方法,或者是axios库等,配合后台API来完成POST请求,并将响应的图片数据设置为`<img>`标签的`src`属性:
```javascript
fetch('your-api-url', {
method: 'POST',
body: yourImageData // 这里是你想要上传的数据
})
.then(response => response.blob())
.then(blob => URL.createObjectURL(blob))
.then(url => document.getElementById('your-image-id').src = url);
```
在这个例子中,你需要确保后端API能接受POST请求并返回适合浏览器使用的图片格式(如JPEG、PNG等)。
相关问题
import os import requests from bs4 import BeautifulSoup # 定义函数,下载图片并保存到指定目录下 def download_img(img_url, path): response = requests.get(img_url) with open(path, 'wb') as f: f.write(response.content) # 论坛模块链接 url = 'https://bbs.zol.com.cn/dcbbs/d15.html' # 发送请求 response = requests.get(url) # 解析页面 soup = BeautifulSoup(response.text, 'html.parser') # 找到所有帖子的链接 post_links = soup.select('.post-td a') # 遍历所有帖子链接 for post_link in post_links: post_url = post_link['href'] post_response = requests.get(post_url) post_soup = BeautifulSoup(post_response.text, 'html.parser') # 找到帖子标题 post_title = post_soup.select('.post-title a')[0].text.strip() # 创建以帖子标题为名字的文件夹 folder_path = os.path.join(os.getcwd(), post_title) if not os.path.exists(folder_path): os.makedirs(folder_path) # 找到帖子中所有图片链接 img_links = post_soup.select('.post-text img') # 遍历所有图片链接,下载并保存到文件夹中 for i, img_link in enumerate(img_links): img_url = img_link['src'] img_path = os.path.join(folder_path, f'{i}.jpg') download_img(img_url, img_path)报错修改C:\ProgramData\Anaconda3\lib\site-packages\urllib3\connection.py:329: SystemTimeWarning: System time is way off (before 2019-01-01). This will probably lead to SSL verification errors warnings.warn(
这个报错是由于你的系统时间设置不正确导致的,可以尝试手动修改系统时间来解决。
如果你使用的是Anaconda环境,可以尝试打开Anaconda Prompt,使用以下命令来更新conda的环境:
```
conda update --all
```
如果还是无法解决问题,可以尝试升级urllib3库到最新版本:
```
pip install --upgrade urllib3
```
如果还有问题,请提供完整报错信息,我会尽力帮助你解决。
vue post请求后,后台返回二进制图片,如何在页面中渲染
在Vue.js中,当你从服务器获取到二进制图片数据时,可以将其转化为Blob或Data URL,然后通过`<img>`标签展示出来。以下是步骤:
1. **处理后端响应**:
在发送POST请求并接收到服务器返回的数据时,检查数据类型。如果是二进制数据,通常会是一个Buffer对象或其他形式的二进制流。
```javascript
axios.post('your-api-endpoint', data).then(response => {
if (response.data instanceof ArrayBuffer) {
// 这里假设 response.data 是一个ArrayBuffer实例
let binaryString = '';
const arrayBufferView = new Uint8Array(response.data);
for (let i = 0; i < arrayBufferView.byteLength; i++) {
binaryString += String.fromCharCode(arrayBufferView[i]);
}
// 现在binaryString就是二进制字符串了
} else {
throw new Error('Invalid response format');
}
});
```
2. **转换为Blob或DataURL**:
转换二进制字符串为Blob对象,然后转成Data URL以便在img标签中显示。
```javascript
const blob = new Blob([binaryString], { type: 'image/jpeg' }); // 根据实际图片格式设置type
const url = URL.createObjectURL(blob); // 创建Data URL
// 图片元素
const imgElement = document.createElement('img');
imgElement.src = url;
imgElement.onload = () => URL.revokeObjectURL(url); // 加载完成后释放资源
document.body.appendChild(imgElement);
```
阅读全文
相关推荐
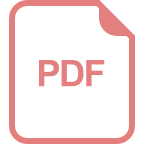
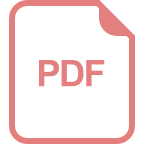
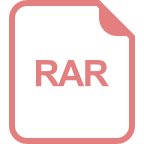
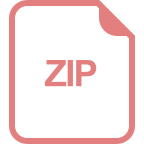
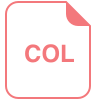
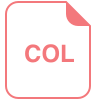
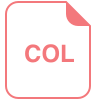









