vite vue3 ts 如何导入api接口
时间: 2024-10-20 15:07:43 浏览: 37
在Vite项目中使用Vue3和TypeScript时,导入API接口通常会涉及以下几个步骤:
1. 安装依赖:首先确保已经安装了`axios`库来处理HTTP请求,以及`@types/axios`提供TypeScript支持。你可以通过运行以下命令安装:
```
npm install axios @types/axios
```
2. 创建接口文件:在src/api目录下创建一个名为`api.ts`的文件,用于声明你的接口类型。例如:
```typescript
// api.ts
export interface User {
id: number;
name: string;
}
export const getUser = async (): Promise<User> => {
// 这里是你实际调用API的代码,例如使用axios.get()
return { id: 1, name: 'John Doe' };
};
```
3. 导入并在组件中使用:在需要使用API的地方,如一个组件内,导入并注入`getUser`函数:
```typescript
// YourComponent.vue
import { ref, onMounted } from 'vue';
import { getUser } from '@/api/api';
export default {
setup() {
const user = ref(null);
onMounted(async () => {
try {
const response = await getUser();
user.value = response as User;
} catch (error) {
console.error('Failed to fetch user', error);
}
});
return {
user,
};
},
};
```
4. 使用接口类型保护:如果API返回的数据结构有变化,可以在接口中添加类型断言(type assertion),防止错误的类型注入:
```typescript
user.value = response as User; // 类型断言
```
阅读全文
相关推荐
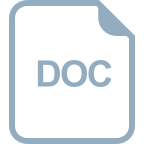
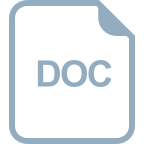
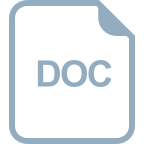
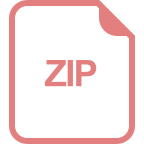
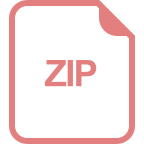
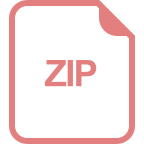
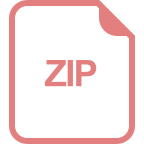











