)某城市天气情况如下,使用Java程序按温度从高到低输出天气信息。要求如下: 使用3个一维数组存储日期、温度和湿度信息; 使用2个二维数组存储日期、温度和湿度信息。
时间: 2024-09-17 10:06:34 浏览: 75
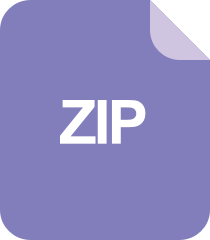
calendarjava源码-A2Temperature:编写一个程序,该程序使用二维数组存储日历年每个月的最高和最低温度。温度将在键盘上输入
在Java中,你可以使用一维数组或者二维数组来存储天气数据,然后通过循环遍历并按照温度从高到低排序输出。这里我将分别解释如何使用这两种方式。
**一维数组的方式**:
```java
// 定义三个一维数组
String[] dates = {"2023-01-01", "2023-01-02", "2023-01-03"}; // 假设这是日期
int[] temperatures = {35, 30, 32}; // 温度
int[] humidities = {60, 70, 65}; // 湿度
// 创建一个新的数组用于存储排序后的索引
int[] sortedIndices = new int[tempatures.length];
for (int i = 0; i < temperatures.length; i++) {
sortedIndices[i] = i;
}
// 对温度数组进行排序
for (int i = 0; i < temperatures.length - 1; i++) {
for (int j = 0; j < temperatures.length - 1 - i; j++) {
if (temperatures[j] > temperatures[j + 1]) {
int temp = temperatures[j];
temperatures[j] = temperatures[j + 1];
temperatures[j + 1] = temp;
int humidity = humidities[j];
humidities[j] = humidities[j + 1];
humidities[j + 1] = humidity;
sortedIndices[j] = j + 1;
sortedIndices[j + 1] = j;
}
}
}
// 根据排序后的索引输出天气信息
for (int i = 0; i < temperatures.length; i++) {
System.out.println("日期:" + dates[sortedIndices[i]] + ", 温度:" + temperatures[i] + ", 湿度:" + humidities[i]);
}
```
**二维数组的方式**:
```java
// 定义两个二维数组
String[][] dateTempsHums = {
{"2023-01-01", 35, 60},
{"2023-01-02", 30, 70},
{"2023-01-03", 32, 65}
};
// 使用自定义Comparator进行排序
List<WeatherData> weatherList = Arrays.asList(dateTempsHums);
Collections.sort(weatherList, Comparator.comparingInt(WeatherData::getTemperature).reversed());
// 自定义WeatherData类
class WeatherData {
String date;
int temperature;
int humidity;
// getters and setters
}
// 输出排序后的天气信息
for (WeatherData data : weatherList) {
System.out.println("日期:" + data.date + ", 温度:" + data.temperature + ", 湿度:" + data.humidity);
}
```
在这个例子中,我们假设了一个`WeatherData`类,并使用了`java.util.Collections.sort()`方法对二维数组进行了排序。
阅读全文
相关推荐
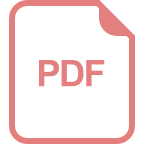
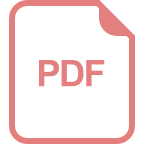


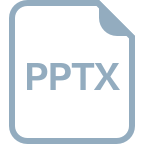
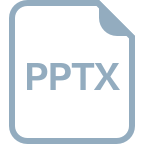
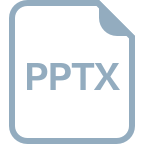
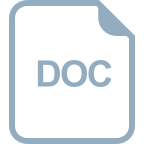
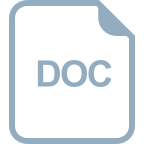
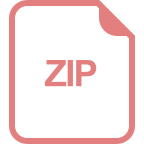
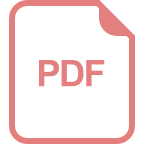
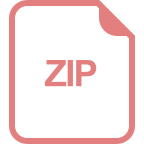
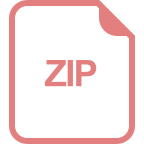
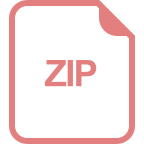
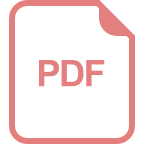
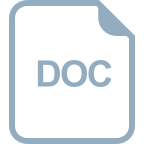
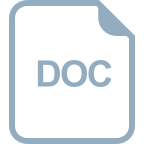