请帮我用c++写一个双人回制对打小游戏,要使用类,有血量攻击力和防御力等
时间: 2024-05-12 07:16:10 浏览: 145
属性,可以进行攻击和防御。
好的,下面是一个基本的双人回合制对打小游戏的C++代码,使用了类和属性。
```
#include <iostream>
#include <string>
using namespace std;
// 定义角色类
class Role {
public:
string name; // 角色名
int hp; // 生命值
int attack; // 攻击力
int defense; // 防御力
Role(string name, int hp, int attack, int defense) {
this->name = name;
this->hp = hp;
this->attack = attack;
this->defense = defense;
}
void attackTarget(Role& target) {
int damage = this->attack - target.defense;
if (damage < 0) {
damage = 0;
}
target.hp -= damage;
cout << this->name << "对" << target.name << "造成了" << damage << "点伤害!" << endl;
cout << target.name << "的生命值剩余" << target.hp << endl;
if (target.hp <= 0) {
cout << target.name << "已经死亡!" << endl;
}
}
void defenseSelf() {
cout << this->name << "使用了防御!" << endl;
this->defense *= 2;
}
};
int main() {
Role player1("Player1", 100, 20, 10);
Role player2("Player2", 100, 18, 12);
int round = 1;
while (player1.hp > 0 && player2.hp > 0) {
cout << "第" << round << "回合开始!" << endl;
cout << player1.name << "的生命值:" << player1.hp << endl;
cout << player2.name << "的生命值:" << player2.hp << endl;
// 玩家1回合
cout << player1.name << "的回合:" << endl;
string input;
do {
cout << "请选择行动(1.攻击 2.防御):";
cin >> input;
} while (input != "1" && input != "2");
if (input == "1") {
player1.attackTarget(player2);
} else if (input == "2") {
player1.defenseSelf();
}
// 玩家2回合
cout << player2.name << "的回合:" << endl;
do {
cout << "请选择行动(1.攻击 2.防御):";
cin >> input;
} while (input != "1" && input != "2");
if (input == "1") {
player2.attackTarget(player1);
} else if (input == "2") {
player2.defenseSelf();
}
round++;
}
if (player1.hp <= 0) {
cout << player2.name << "获胜!" << endl;
} else if (player2.hp <= 0) {
cout << player1.name << "获胜!" << endl;
}
return 0;
}
```
希望这个代码能够满足你的需求!
阅读全文
相关推荐
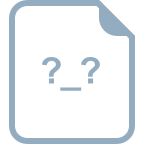
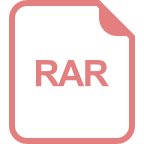
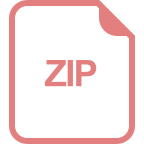
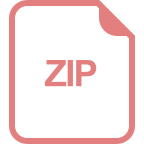
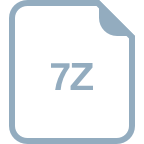
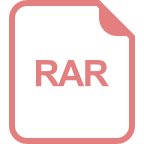
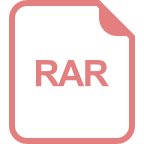
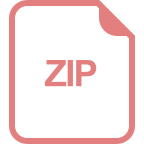