一个listview设置二级列表 父列表一个对应多个子列表 使用list数据 demo
时间: 2024-03-11 07:50:16 浏览: 88
好的,这里提供一个简单的示例代码:
```java
// 父列表数据
List<String> parentList = new ArrayList<>();
parentList.add("父列表1");
parentList.add("父列表2");
parentList.add("父列表3");
// 子列表数据
Map<String, List<String>> childMap = new HashMap<>();
List<String> childList1 = new ArrayList<>();
childList1.add("子列表1-1");
childList1.add("子列表1-2");
childList1.add("子列表1-3");
childMap.put("父列表1", childList1);
List<String> childList2 = new ArrayList<>();
childList2.add("子列表2-1");
childList2.add("子列表2-2");
childMap.put("父列表2", childList2);
List<String> childList3 = new ArrayList<>();
childList3.add("子列表3-1");
childList3.add("子列表3-2");
childList3.add("子列表3-3");
childMap.put("父列表3", childList3);
// 构造适配器
ExpandableListAdapter adapter = new ExpandableListAdapter(parentList, childMap);
listView.setAdapter(adapter);
```
其中,`ExpandableListAdapter` 是自定义的一个适配器类,实现了 `ExpandableListAdapter` 接口,可以根据自己的需求进行定制。在适配器中,需要实现以下几个方法:
```java
@Override
public int getGroupCount() {
return parentList.size();
}
@Override
public int getChildrenCount(int groupPosition) {
String parent = parentList.get(groupPosition);
List<String> child = childMap.get(parent);
return child.size();
}
@Override
public Object getGroup(int groupPosition) {
return parentList.get(groupPosition);
}
@Override
public Object getChild(int groupPosition, int childPosition) {
String parent = parentList.get(groupPosition);
List<String> child = childMap.get(parent);
return child.get(childPosition);
}
@Override
public long getGroupId(int groupPosition) {
return groupPosition;
}
@Override
public long getChildId(int groupPosition, int childPosition) {
return childPosition;
}
@Override
public boolean hasStableIds() {
return true;
}
@Override
public View getGroupView(int groupPosition, boolean isExpanded, View convertView, ViewGroup parent) {
// 构造父列表项视图
return parentView;
}
@Override
public View getChildView(int groupPosition, int childPosition, boolean isLastChild, View convertView, ViewGroup parent) {
// 构造子列表项视图
return childView;
}
@Override
public boolean isChildSelectable(int groupPosition, int childPosition) {
return true;
}
```
其中,`getGroupCount()` 返回父列表项的个数,`getChildrenCount(int groupPosition)` 返回指定父列表项下的子列表项的个数,`getGroup(int groupPosition)` 返回指定父列表项的数据,`getChild(int groupPosition, int childPosition)` 返回指定父列表项和子列表项的数据,`getGroupId(int groupPosition)` 和 `getChildId(int groupPosition, int childPosition)` 返回指定父列表项和子列表项的 ID,`hasStableIds()` 返回是否具有稳定的 ID,`getGroupView()` 构造父列表项的视图,`getChildView()` 构造子列表项的视图,`isChildSelectable()` 返回子列表项是否可选。
阅读全文
相关推荐

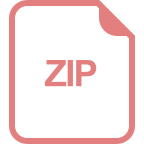
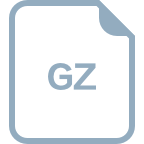
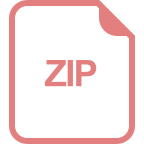
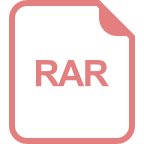
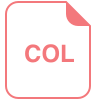

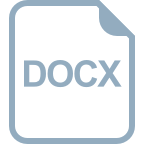
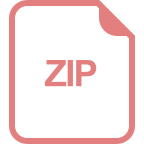