编写程序,输入一个字符串,过滤掉所有非小写字母,得到由小写字母组成的字符串并输出。
时间: 2024-03-08 13:49:05 浏览: 54
好的,以下是 Python 的代码实现:
```python
s = input("请输入一个字符串:")
# 过滤非小写字母
filtered = ""
for c in s:
if c.islower():
filtered += c
print("过滤后的字符串为:", filtered)
```
这段代码首先让用户输入一个字符串,然后遍历字符串中的每个字符,如果该字符是小写字母,就将其添加到 `filtered` 字符串中。最后输出过滤后的字符串即可。
相关问题
编写程序,输入一个字符串,过滤掉所有非小写字母,得到由小写字母组成的字符串并输出。用C语言
好的,以下是C语言的代码实现:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char s[100];
printf("请输入一个字符串:");
scanf("%s", s);
// 过滤非小写字母
char filtered[100];
int j = 0;
for (int i = 0; s[i] != '\0'; i++) {
if (islower(s[i])) {
filtered[j++] = s[i];
}
}
filtered[j] = '\0';
printf("过滤后的字符串为:%s\n", filtered);
return 0;
}
```
这段代码首先定义了一个字符数组 `s`,用于存储用户输入的字符串。然后使用 `scanf` 函数从标准输入中读取用户输入的字符串。
接下来,我们定义了另一个字符数组 `filtered`,用于存储过滤后的字符串。我们使用一个循环遍历输入字符串中的每个字符,如果该字符是小写字母,则将其加入 `filtered` 中。
最后,我们在 `filtered` 的末尾添加一个空字符 `\0`,以表示字符串的结尾,并使用 `printf` 函数输出过滤后的字符串。
注意,这段代码中使用了 `<ctype.h>` 头文件中的 `islower` 函数,用于判断一个字符是否为小写字母。
编写程序,输入一个字符串,将其中的小写字母修改为大写字母 输出该字符串
您可以使用 Python 编写以下程序来将输入字符串中的小写字母修改为大写字母:
```python
string = input("请输入一个字符串:") # 获取用户输入的字符串
upper_string = string.upper() # 将字符串中的小写字母修改为大写字母
print("修改后的字符串为:", upper_string) # 输出修改后的字符串
```
这段代码中,我们首先使用 `input()` 函数获取用户输入的字符串,并将其保存在变量 `string` 中。然后,我们使用字符串的 `upper()` 方法将其中的小写字母修改为大写字母,并将结果保存在变量 `upper_string` 中。最后,我们使用 `print()` 函数输出修改后的字符串。
例如,如果用户输入了字符串 "Hello, world!", 则程序会输出 "HELLO, WORLD!"。
阅读全文
相关推荐
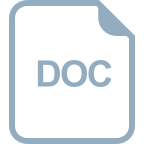
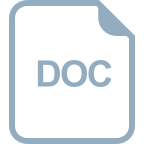
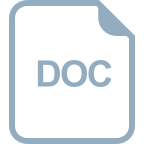













