编程实现 生产者-消费者(仓库)程序java
时间: 2024-01-13 17:03:40 浏览: 72
生产者-消费者问题是一个经典的并发编程问题,下面是一个简单的 Java 实现:
```java
import java.util.LinkedList;
public class ProducerConsumer {
private LinkedList<Integer> buffer = new LinkedList<>();
private int maxSize = 5;
public void produce() throws InterruptedException {
int value = 0;
while (true) {
synchronized (this) {
while (buffer.size() == maxSize) {
wait();
}
System.out.println("生产者生产了:" + value);
buffer.add(value++);
notify();
}
Thread.sleep(1000); // 生产者生产完之后休眠1秒
}
}
public void consume() throws InterruptedException {
while (true) {
synchronized (this) {
while (buffer.isEmpty()) {
wait();
}
int value = buffer.removeFirst();
System.out.println("消费者消费了:" + value);
notify();
}
Thread.sleep(1000); // 消费者消费完之后休眠1秒
}
}
public static void main(String[] args) {
ProducerConsumer pc = new ProducerConsumer();
Thread producer = new Thread(() -> {
try {
pc.produce();
} catch (InterruptedException e) {
e.printStackTrace();
}
});
Thread consumer = new Thread(() -> {
try {
pc.consume();
} catch (InterruptedException e) {
e.printStackTrace();
}
});
producer.start();
consumer.start();
}
}
```
在这个程序中,使用 `LinkedList` 来作为仓库,`maxSize` 指定了仓库的最大大小。生产者线程不断地生产数据并放入仓库,如果仓库满了,则生产者线程进入等待状态。消费者线程不断地从仓库中取出数据并消费,如果仓库为空,则消费者线程进入等待状态。
在 `produce()` 和 `consume()` 方法中,使用了 `synchronized` 关键字来保证线程安全性。在生产者线程生产完数据或者消费者线程消费完数据之后,调用 `notify()` 方法来通知另一个线程可以继续执行。在生产者线程和消费者线程中都使用了 `wait()` 方法来进入等待状态,等待另一个线程的通知。
最后,在 `main()` 方法中创建了一个生产者线程和一个消费者线程,并启动它们。
阅读全文
相关推荐
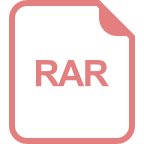
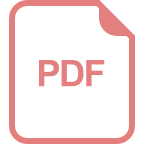
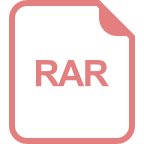
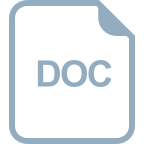
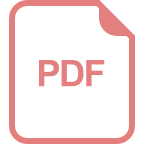
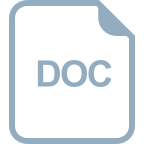
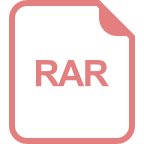
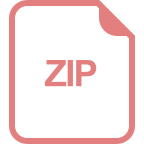
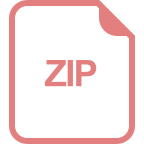
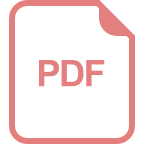
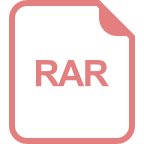
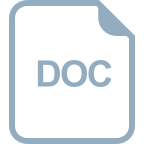
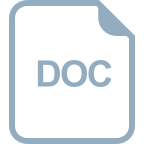
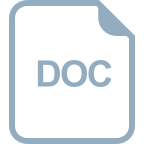
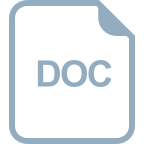
