使用python实现一下功能:使用树莓派读取PCF8591模块的温度传感器和光敏传感器信息
时间: 2023-07-20 11:45:58 浏览: 139
好的,下面是实现步骤:
1. 确保树莓派已经连接了PCF8591模块,并且已经安装好了smbus2库。
2. 导入需要的库:
```python
import smbus2
import time
```
3. 定义PCF8591模块的地址和传感器通道:
```python
# PCF8591模块地址
address = 0x48
# 传感器通道
temp_channel = 0
light_channel = 1
```
4. 定义读取传感器数值的函数:
```python
def read_sensor(channel):
with smbus2.SMBus(1) as bus:
# 向PCF8591模块写入要读取的通道
bus.write_byte(address, channel)
# 等待转换完成
time.sleep(0.5)
# 读取转换结果
value = bus.read_byte(address)
return value
```
5. 调用函数读取温度传感器和光敏传感器的数值:
```python
# 读取温度传感器数值
temp_value = read_sensor(temp_channel)
# 读取光敏传感器数值
light_value = read_sensor(light_channel)
```
6. 打印数值:
```python
print("温度传感器数值:", temp_value)
print("光敏传感器数值:", light_value)
```
完整代码如下:
```python
import smbus2
import time
# PCF8591模块地址
address = 0x48
# 传感器通道
temp_channel = 0
light_channel = 1
def read_sensor(channel):
with smbus2.SMBus(1) as bus:
# 向PCF8591模块写入要读取的通道
bus.write_byte(address, channel)
# 等待转换完成
time.sleep(0.5)
# 读取转换结果
value = bus.read_byte(address)
return value
# 读取温度传感器数值
temp_value = read_sensor(temp_channel)
# 读取光敏传感器数值
light_value = read_sensor(light_channel)
print("温度传感器数值:", temp_value)
print("光敏传感器数值:", light_value)
```
阅读全文
相关推荐
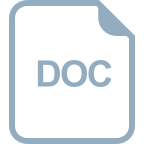
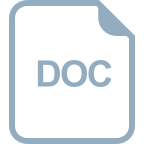
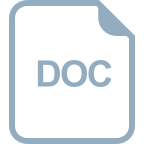
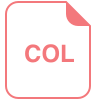

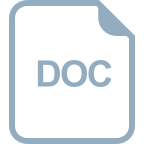
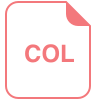
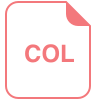
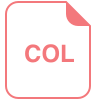
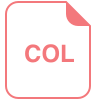
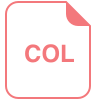
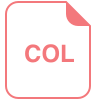
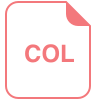

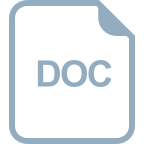
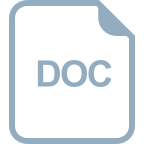
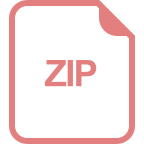
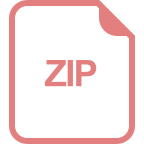