springboot中如何实现kafka消费者发起webclient网络请求
时间: 2023-03-05 11:33:35 浏览: 146
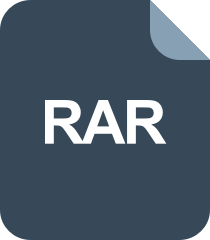
springboot 基于spring-kafka动态创建kafka消费者
在Spring Boot中实现Kafka消费者发起WebClient网络请求可以通过以下步骤实现:
1. 引入Kafka和WebFlux依赖。可以在项目的`pom.xml`文件中添加以下依赖:
```
<dependency>
<groupId>org.springframework.kafka</groupId>
<artifactId>spring-kafka</artifactId>
<version>${spring.kafka.version}</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-webflux</artifactId>
<version>${spring.boot.version}</version>
</dependency>
```
2. 创建Kafka消费者。可以使用Spring Kafka提供的`@KafkaListener`注解创建一个消费者,用于监听特定主题的消息。例如:
```
@KafkaListener(topics = "test-topic")
public void processMessage(String message) {
// 处理Kafka消息
}
```
3. 在消费者中发起WebClient网络请求。可以使用Spring WebFlux提供的`WebClient`类发起网络请求。例如:
```
@Autowired
private WebClient webClient;
@KafkaListener(topics = "test-topic")
public void processMessage(String message) {
Mono<String> result = webClient.get()
.uri("https://example.com")
.retrieve()
.bodyToMono(String.class);
// 处理网络请求结果
}
```
在上面的示例中,`WebClient`被注入到了消费者中,然后使用`WebClient`发起一个GET请求并将响应体解析为一个字符串。你可以根据实际需求修改请求的URL、请求方法、请求体等。
需要注意的是,WebClient默认使用的是非阻塞式I/O,这意味着网络请求将会异步地执行,不会阻塞当前线程。如果需要使用阻塞式I/O,可以在创建`WebClient`时指定使用`ExchangeStrategies#withDefaults`的阻塞式I/O策略,例如:
```
@Bean
public WebClient webClient() {
return WebClient.builder()
.exchangeStrategies(ExchangeStrategies.builder()
.codecs(configurer -> configurer.defaultCodecs().maxInMemorySize(16 * 1024 * 1024))
.build())
.build();
}
```
这样,就可以在Kafka消费者中发起WebClient网络请求了。
阅读全文
相关推荐
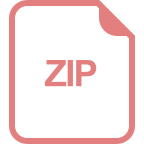
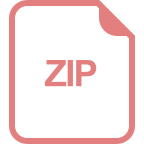

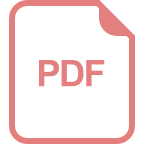
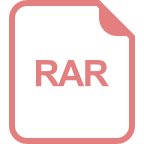
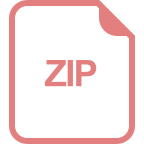
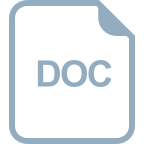

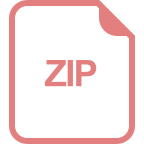
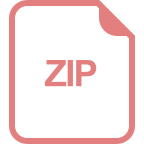
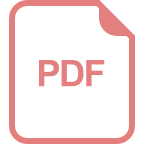
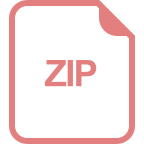
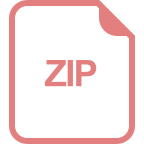
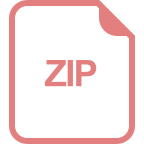
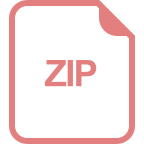
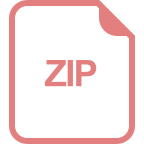
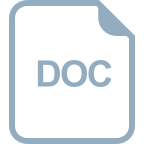
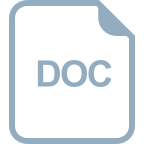
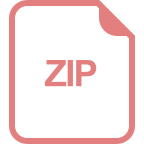