Java 设计模式跟使用场景代码
时间: 2023-10-14 10:06:58 浏览: 145
Java 中有许多种设计模式,每种模式都有不同的使用场景。以下是一些常见的设计模式及其使用场景示例代码:
1. 单例模式:用于保证一个类只有一个实例,并提供一个访问该实例的全局访问点。
```java
public class Singleton {
private static Singleton instance;
private Singleton() {}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
// 使用示例
Singleton obj = Singleton.getInstance();
```
2. 工厂方法模式:定义一个用于创建对象的接口,让子类决定实例化哪一个类。
```java
public interface AnimalFactory {
public Animal createAnimal();
}
public class DogFactory implements AnimalFactory {
public Animal createAnimal() {
return new Dog();
}
}
public class CatFactory implements AnimalFactory {
public Animal createAnimal() {
return new Cat();
}
}
// 使用示例
AnimalFactory factory = new DogFactory();
Animal animal = factory.createAnimal();
```
3. 抽象工厂模式:提供一个创建一系列相关或相互依赖对象的接口,而无需指定它们具体的类。
```java
public interface AbstractFactory {
public Animal createAnimal();
public Plant createPlant();
}
public class LandFactory implements AbstractFactory {
public Animal createAnimal() {
return new Dog();
}
public Plant createPlant() {
return new Tree();
}
}
public class SeaFactory implements AbstractFactory {
public Animal createAnimal() {
return new Fish();
}
public Plant createPlant() {
return new Seaweed();
}
}
// 使用示例
AbstractFactory factory = new LandFactory();
Animal animal = factory.createAnimal();
Plant plant = factory.createPlant();
```
4. 观察者模式:定义对象间的一种一对多的依赖关系,当一个对象的状态发生改变时,所有依赖它的对象都得到通知并自动更新。
```java
public interface Observer {
public void update();
}
public class Subject {
private List<Observer> observers = new ArrayList<Observer>();
public void addObserver(Observer observer) {
observers.add(observer);
}
public void removeObserver(Observer observer) {
observers.remove(observer);
}
public void notifyObservers() {
for (Observer observer : observers) {
observer.update();
}
}
}
public class ConcreteObserver implements Observer {
public void update() {
System.out.println("Subject has been updated");
}
}
// 使用示例
Subject subject = new Subject();
Observer observer = new ConcreteObserver();
subject.addObserver(observer);
subject.notifyObservers();
```
以上是一些常见的设计模式及其使用场景示例代码,当然还有许多其他的设计模式和使用场景。在实际的开发中,根据具体的需求来选择合适的设计模式非常重要。
阅读全文
相关推荐





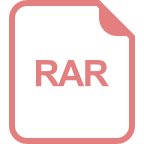

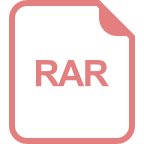

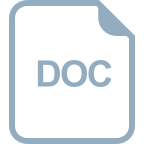



