做三个类型的在线考试题(每个题100分),题一为单选题,题二为多选题,题三为填空题,每题答完以后有提交按钮显示该题是否正确,显示客户端提交的答案,以及该题的得分,题目自拟,最后做一个交卷的按钮,点击以后显示客户端总共答对多少题,答错多少题以及最后得分
时间: 2024-05-16 16:14:19 浏览: 216
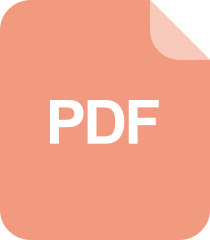
OExam试题设置详解
好的,这是一个比较复杂的需求,需要涉及到前后端的交互和数据存储,以下是一个简单的实现过程:
1. 后端设计
首先要设计好后端的 API 接口,包括获取题目、提交答案、计算分数等功能。可以使用 Django 或 Flask 等框架来搭建后端。
下面是一个简单的 Django 后端示例:
```python
from django.shortcuts import render
from django.http import JsonResponse
# 单选题列表
single_choices = [
{
'id': 1,
'question': '以下哪个不是 Python 的数据类型?',
'choices': [
'int',
'double',
'str',
'bool'
],
'answer': 'double',
'score': 100
},
{
'id': 2,
'question': '以下哪个函数可以将字符串转换为整数?',
'choices': [
'int()',
'float()',
'str()',
'bool()'
],
'answer': 'int()',
'score': 100
},
# ...
]
# 多选题列表
multiple_choices = [
{
'id': 1,
'question': '以下哪些语言是 Web 开发中常用的?',
'choices': [
'Python',
'Java',
'JavaScript',
'C++'
],
'answer': ['Python', 'Java', 'JavaScript'],
'score': 100
},
{
'id': 2,
'question': '以下哪些数据库是关系型数据库?',
'choices': [
'MySQL',
'MongoDB',
'Redis',
'Oracle'
],
'answer': ['MySQL', 'Oracle'],
'score': 100
},
# ...
]
# 填空题列表
fill_blank_questions = [
{
'id': 1,
'question': 'Python 中用来表示“真”的关键字是__1__,用来表示“假”的关键字是__2__。',
'answer': ['True', 'False'],
'score': 100
},
{
'id': 2,
'question': 'Python 中可以通过__1__语句来定义一个函数,通过__2__语句来调用函数。',
'answer': ['def', 'return'],
'score': 100
},
# ...
]
def get_single_choice(request, id):
# 根据 ID 获取单选题
for choice in single_choices:
if choice['id'] == id:
return JsonResponse(choice)
return JsonResponse({'error': 'not found'})
def get_multiple_choice(request, id):
# 根据 ID 获取多选题
for choice in multiple_choices:
if choice['id'] == id:
return JsonResponse(choice)
return JsonResponse({'error': 'not found'})
def get_fill_blank_question(request, id):
# 根据 ID 获取填空题
for question in fill_blank_questions:
if question['id'] == id:
return JsonResponse(question)
return JsonResponse({'error': 'not found'})
def submit_answer(request):
# 获取客户端提交的答案和题目类型
answer = request.POST.get('answer')
question_type = request.POST.get('type')
# 根据题目类型计算分数
if question_type == 'single_choice':
# ...
elif question_type == 'multiple_choice':
# ...
elif question_type == 'fill_blank':
# ...
# 返回分数
return JsonResponse({'score': score})
```
2. 前端设计
前端需要设计好界面,包括题目、选项、答案输入框、提交按钮等。可以使用 Vue 或 React 等框架来搭建前端。
下面是一个简单的 Vue 前端示例:
```vue
<template>
<div>
<!-- 单选题 -->
<div v-if="questionType === 'single_choice'">
<p>{{ question }}</p>
<div v-for="(choice, index) in choices" :key="index">
<input type="radio" :value="choice" v-model="answer" @change="checkAnswer">
<label>{{ choice }}</label>
</div>
</div>
<!-- 多选题 -->
<div v-if="questionType === 'multiple_choice'">
<p>{{ question }}</p>
<div v-for="(choice, index) in choices" :key="index">
<input type="checkbox" :value="choice" v-model="answer" @change="checkAnswer">
<label>{{ choice }}</label>
</div>
</div>
<!-- 填空题 -->
<div v-if="questionType === 'fill_blank'">
<p v-html="question"></p>
<input v-for="(blank, index) in blanks" :key="index" type="text" v-model="answer[index]" @change="checkAnswer">
</div>
<p v-if="showResult">答案:{{ result.answer }},得分:{{ result.score }}</p>
<button v-if="showSubmit" @click="submitAnswer">提交</button>
<button v-if="showNext" @click="nextQuestion">下一题</button>
<button v-if="showFinish" @click="finishExam">交卷</button>
</div>
</template>
<script>
export default {
data() {
return {
question: '',
choices: [],
blanks: [],
answer: '',
result: {},
questionType: '',
showResult: false,
showSubmit: false,
showNext: false,
showFinish: false
};
},
methods: {
// 获取题目
async getQuestion(id) {
try {
const response = await fetch(`/api/question/${id}`);
const data = await response.json();
this.question = data.question;
this.choices = data.choices;
this.blanks = Array(data.answer.length).fill('');
this.result = {};
this.questionType = id < 3 ? 'single_choice' : id < 6 ? 'multiple_choice' : 'fill_blank';
this.showResult = false;
this.showSubmit = true;
this.showNext = false;
this.showFinish = false;
} catch (error) {
console.error(error);
}
},
// 检查答案
async checkAnswer() {
try {
const response = await fetch('/api/submit', {
method: 'POST',
body: JSON.stringify({
answer: this.answer,
type: this.questionType
}),
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
this.result = data;
this.showResult = true;
this.showSubmit = false;
this.showNext = true;
this.showFinish = false;
} catch (error) {
console.error(error);
}
},
// 下一题
nextQuestion() {
this.getQuestion(this.questionId + 1);
},
// 完成考试
finishExam() {
alert(`总共答对 ${this.correctCount} 题,答错 ${this.wrongCount} 题,得分 ${this.score} 分`);
}
},
computed: {
// 计算总分
score() {
return this.correctCount * 100;
},
// 计算答对的题目数量
correctCount() {
// ...
},
// 计算答错的题目数量
wrongCount() {
// ...
}
},
mounted() {
this.getQuestion(1);
}
};
</script>
```
3. 数据存储
可以使用 SQLite 或 MySQL 等数据库来存储题目和答案,也可以使用 JSON 文件来存储。如果使用数据库,需要在后端代码中添加相应的数据库连接和查询语句。
4. 部署
最后需要将后端和前端部署到服务器上,可以使用 Nginx 或 Apache 等 Web 服务器来搭建。可以使用 Docker 来简化部署过程。
阅读全文
相关推荐
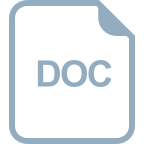
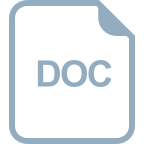

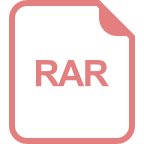
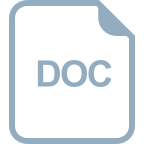
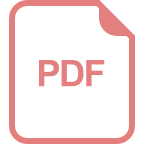
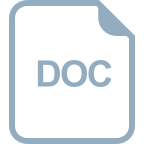
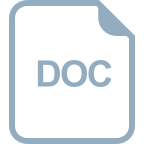
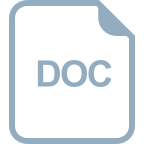
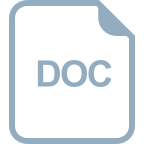
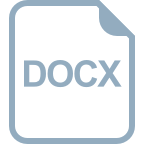
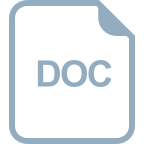
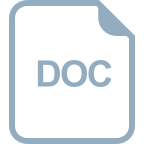
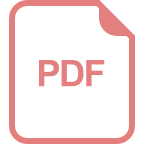
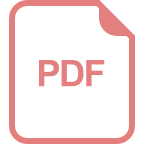
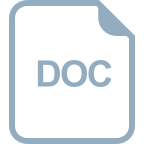
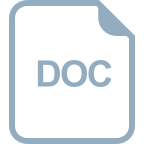
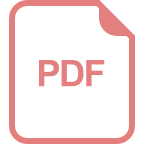