在linux中用c语言实现复制和移动文件
时间: 2023-06-14 11:06:08 浏览: 125
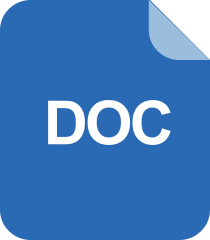
Linux下C语言实现文件拷贝

复文件:
```c
#include <stdio.h>
#include <stdlib.h>
int main() {
FILE *sourceFile, *destFile;
char sourcePath[100], destPath[100], ch;
printf("Enter source file path: ");
scanf("%s", sourcePath);
printf("Enter destination file path: ");
scanf("%s", destPath);
// Open source file in read mode
sourceFile = fopen(sourcePath, "r");
if (sourceFile == NULL) {
printf("Unable to open source file!");
exit(EXIT_FAILURE);
}
// Open destination file in write mode
destFile = fopen(destPath, "w");
if (destFile == NULL) {
printf("Unable to create destination file!");
exit(EXIT_FAILURE);
}
// Copy file contents character by character
while ((ch = fgetc(sourceFile)) != EOF) {
fputc(ch, destFile);
}
printf("File copied successfully.\n");
// Close files to release resources
fclose(sourceFile);
fclose(destFile);
return 0;
}
```
移动文件:
```c
#include <stdio.h>
#include <stdlib.h>
int main() {
char sourcePath[100], destPath[100];
printf("Enter source file path: ");
scanf("%s", sourcePath);
printf("Enter destination file path: ");
scanf("%s", destPath);
// Use rename() function to move file
if(rename(sourcePath, destPath) == 0) {
printf("File moved successfully.\n");
} else {
printf("Unable to move file.\n");
}
return 0;
}
```
以上代码均适用于 Linux 系统。
阅读全文
相关推荐
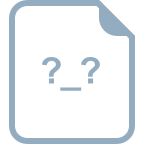
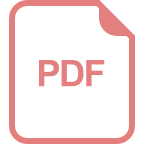
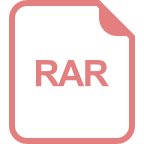
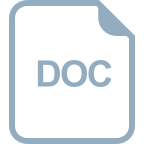
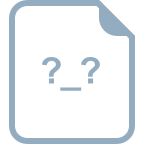
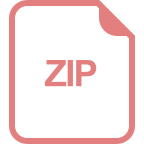
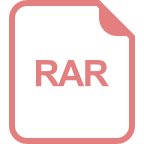
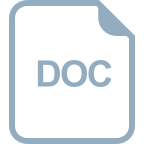
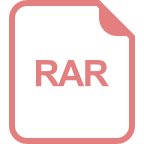
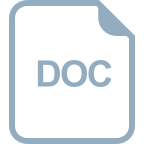
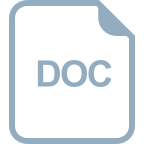
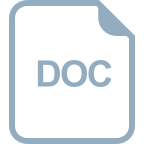
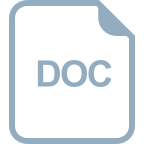
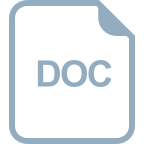
