springboot 读取doc文件
时间: 2023-10-09 12:05:33 浏览: 103
可以使用Apache POI库来读取和操作Microsoft Office文件(包括doc文件)。使用POI库需要添加相应的依赖,例如:
```xml
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.1.0</version>
</dependency>
```
接下来,可以使用POI提供的API来读取doc文件中的内容。具体方法可以参考POI官方文档和示例代码。
相关问题
springboot 读取doc文件内容
您可以使用Apache POI库来读取DOC文件的内容。您需要添加以下依赖项到您的项目中:
```
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.1.2</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>4.1.2</version>
</dependency>
```
然后,您可以使用以下代码来读取DOC文件的内容:
```
FileInputStream fis = new FileInputStream("yourdocfile.doc");
XWPFDocument document = new XWPFDocument(fis);
List<XWPFParagraph> paragraphs = document.getParagraphs();
for (XWPFParagraph para : paragraphs) {
String text = para.getText();
//处理每个段落的文本
}
fis.close();
```
这将读取DOC文件中的每个段落文本并将其放入一个字符串中,您可以在此处理其中的文本。
springboot 上传doc文件并读取doc文件 代码
非常感谢您的提问!以下是一个简单的示例,演示如何使用Spring Boot上传一个doc文件,并读取它:
```
@RestController
public class FileUploadController {
@PostMapping("/uploadFile")
public String uploadFile(@RequestParam("file") MultipartFile file) {
if (file.isEmpty()) {
return "请选择一个文件!";
}
try {
// 文件存储位置
String filePath = "D:/uploads/";
byte[] bytes = file.getBytes();
Path path = Paths.get(filePath + file.getOriginalFilename());
Files.write(path, bytes);
return "文件上传成功!";
} catch (IOException e) {
e.printStackTrace();
return "文件上传失败!";
}
}
@GetMapping("/readDocFile/{fileName}")
public String readDocFile(@PathVariable("fileName") String fileName) {
try {
// 文件读取位置
String filePath = "D:/uploads/";
FileInputStream inputStream = new FileInputStream(filePath + fileName);
XWPFDocument document = new XWPFDocument(inputStream);
XWPFWordExtractor extractor = new XWPFWordExtractor(document);
String text = extractor.getText();
return text;
} catch (IOException e) {
e.printStackTrace();
return "文件读取失败!";
}
}
}
```
在上面的代码中,我们定义了一个名为`FileUploadController`的Spring Boot控制器,它包含两个路由:`/uploadFile`和`/readDocFile`。
`/uploadFile`路由接受一个名为`file`的文件参数,并将该文件保存到我们指定的路径中。如果文件为空,将返回一个错误消息。否则,我们将使用Java的`Files.write()`方法将文件写入磁盘。
`/readDocFile`路由将接受一个名为`fileName`的参数,并尝试将指定的doc文件读取到内存中。在此示例中,我们使用Apache POI库来实现此功能。`XWPFDocument`类用于解析doc文件的内容,`XWPFWordExtractor`类用于提取文本数据。
总的来说,这是一个非常简单的示例,但它演示了如何使用Spring Boot上传和读取doc文件。请注意,在实际生产环境中,您需要进行更多的错误处理、安全性检查和性能优化。
阅读全文
相关推荐
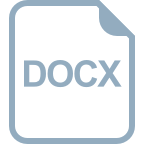
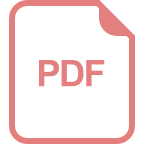



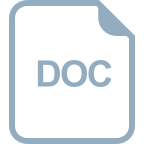
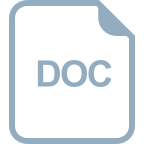
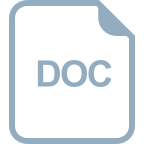
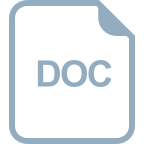
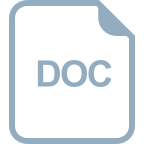
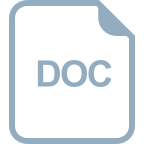



