用Java实现mqTT服务器
时间: 2024-05-01 12:16:53 浏览: 11
MQTT是一种轻量级的发布/订阅协议,可在低带宽和不可靠网络上进行通信。以下是使用Java实现MQTT服务器的基本步骤:
1. 安装Eclipse Mosquitto服务器:Eclipse Mosquitto是一个开源的MQTT服务器,可以在Windows、Linux和Mac OS X平台上运行。从官方网站下载并安装Mosquitto服务器。
2. 编写Java程序:使用Java编写MQTT服务器程序,可以使用Paho MQTT客户端库来实现。该库提供了Java语言的MQTT客户端,可以轻松地与Mosquitto服务器进行通信。
3. 创建MQTT连接:使用Paho MQTT客户端库,创建一个MQTT客户端连接到Mosquitto服务器。连接时需要指定MQTT服务器的地址和端口号。
4. 发布和订阅消息:使用Paho MQTT客户端库,可以轻松地发布和订阅MQTT消息。发布消息时需要指定主题和消息内容,订阅消息时需要指定主题和回调函数。
5. 处理MQTT消息:当有新的MQTT消息到达时,Paho MQTT客户端库将调用回调函数来处理消息。在回调函数中,可以处理收到的消息,并根据需要采取相应的操作。
下面是一个简单的Java程序,用于连接到MQTT服务器、发布和订阅消息:
```java
import org.eclipse.paho.client.mqttv3.*;
import org.eclipse.paho.client.mqttv3.persist.MemoryPersistence;
public class MqttServer {
public static void main(String[] args) {
String broker = "tcp://localhost:1883";
String clientId = "JavaServer";
MemoryPersistence persistence = new MemoryPersistence();
try {
MqttClient client = new MqttClient(broker, clientId, persistence);
// Connect to the MQTT server
client.connect();
// Subscribe to the "test" topic
client.subscribe("test", new MqttCallback() {
public void connectionLost(Throwable cause) {
// Called when the connection to the MQTT server is lost
}
public void messageArrived(String topic, MqttMessage message) throws Exception {
// Called when a new message arrives on the subscribed topic
}
public void deliveryComplete(IMqttDeliveryToken token) {
// Called when a message has been successfully delivered to the MQTT server
}
});
// Publish a message to the "test" topic
String content = "Hello, world!";
MqttMessage message = new MqttMessage(content.getBytes());
message.setQos(0);
client.publish("test", message);
// Disconnect from the MQTT server
client.disconnect();
} catch (MqttException e) {
e.printStackTrace();
}
}
}
```
这个程序连接到本地Mosquitto服务器,订阅名为“test”的主题,并发布一个消息到该主题。当有新的消息到达时,将调用回调函数来处理消息。您可以在回调函数中添加自己的代码,来处理MQTT消息。
请注意,本示例程序仅用于演示目的,实际使用中需要根据需要修改。例如,您可能需要指定其他的MQTT服务器地址和端口号,并更改订阅的主题和发布的消息内容。
相关推荐
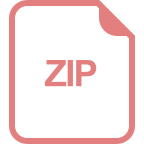
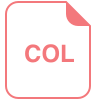
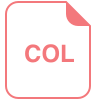
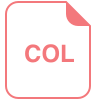
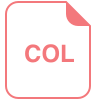
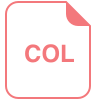









