keil4C语言调用汇编求阶乘和
时间: 2024-09-26 12:08:33 浏览: 42
在Keil uVision 4集成开发环境中,C语言可以方便地调用汇编代码来实现特定功能,比如计算阶乘。首先,你需要编写一段汇编代码来完成阶乘的计算,通常这会在低级优化方面提供优势。这里是一个简化的例子:
**C语言部分:**
```c
#include "stdio.h"
// 函数声明
extern unsigned long long factorial(unsigned int n);
int main(void) {
unsigned int num;
printf("Enter a non-negative integer: ");
scanf("%u", &num);
unsigned long long result = factorial(num);
printf("The factorial of %d is %llu\n", num, result);
return 0;
}
// 汇编函数声明 (假设名字是calc_factorial)
__asm("calc_factorial:")
```
**汇编部分 (示例,使用ARM Cortex-M处理器):**
```assembly
calc_factorial:
// 汇编实现求阶乘的代码
; 使用循环和移位操作来避免溢出
push {lr} @ Save the lr register
mov r0, #1 @ Initialize result to 1
mov r1, #0 @ Initialize counter to 0
factorial_loop:
cmp r1, r0 @ Compare counter with current result
beq end_loop @ If equal, we're done
mul r0, r0, r1 @ Multiply result by counter
add r1, r1, #1 @ Increment counter
b factorial_loop @ Go back to the loop
end_loop:
pop {pc} @ Restore the lr and return to C function caller
```
在汇编代码完成后,需要将它添加到项目中的某个汇编源文件中,并确保正确链接到主C程序。
注意:实际编译过程可能会依赖于你所使用的Keil工具集的具体设置以及目标硬件平台。
阅读全文
相关推荐



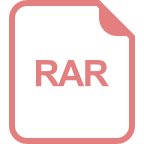










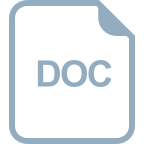