python 实现最小二乘法影像匹配算法
时间: 2023-06-12 07:04:28 浏览: 255
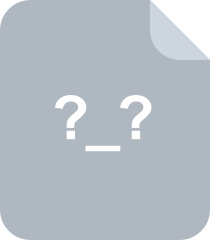
最小二乘影像匹配

最小二乘法影像匹配算法是一种常用的遥感影像处理方法。它的基本思想是通过对两幅遥感影像进行配准,找到它们之间的变换关系,从而实现影像的叠加或融合。下面是Python实现最小二乘法影像匹配算法的步骤:
1. 读取待配准图像和参考图像,将它们转换为灰度图像。
```python
import numpy as np
import cv2
# 读取待配准图像和参考图像
img1 = cv2.imread('img1.jpg')
img2 = cv2.imread('img2.jpg')
# 将图像转换为灰度图像
gray1 = cv2.cvtColor(img1, cv2.COLOR_BGR2GRAY)
gray2 = cv2.cvtColor(img2, cv2.COLOR_BGR2GRAY)
```
2. 提取图像特征点。
```python
# 创建ORB特征检测器
orb = cv2.ORB_create()
# 在图像中提取特征点和特征描述符
kp1, des1 = orb.detectAndCompute(gray1, None)
kp2, des2 = orb.detectAndCompute(gray2, None)
```
3. 对特征点进行匹配。
```python
# 创建暴力匹配器
bf = cv2.BFMatcher(cv2.NORM_HAMMING, crossCheck=True)
# 对特征点进行匹配
matches = bf.match(des1, des2)
# 将匹配结果按照距离从小到大排序
matches = sorted(matches, key=lambda x:x.distance)
```
4. 根据匹配结果计算变换矩阵。
```python
# 取前10个最佳匹配点
good_matches = matches[:10]
# 提取匹配点的坐标
src_pts = np.float32([ kp1[m.queryIdx].pt for m in good_matches ]).reshape(-1,1,2)
dst_pts = np.float32([ kp2[m.trainIdx].pt for m in good_matches ]).reshape(-1,1,2)
# 计算变换矩阵
M, mask = cv2.findHomography(src_pts, dst_pts, cv2.RANSAC,5.0)
```
5. 对待配准图像进行变换。
```python
# 对待配准图像进行变换
aligned_img = cv2.warpPerspective(img1, M, (img2.shape[1],img2.shape[0]))
# 显示结果
cv2.imshow('aligned_img', aligned_img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这样,我们就完成了最小二乘法影像匹配算法的Python实现。
阅读全文
相关推荐
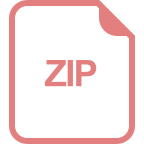
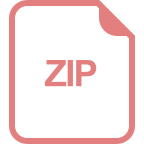
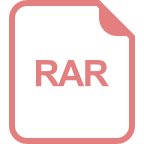
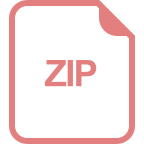
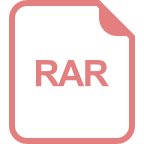
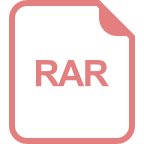
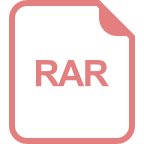
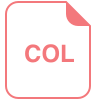
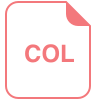
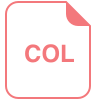
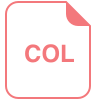
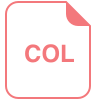
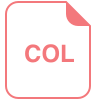
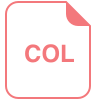
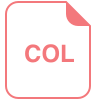
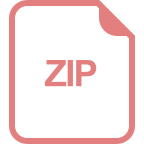