写一段Android studio的代码,写一个登录页面加上记事本备忘录
时间: 2024-02-20 12:01:53 浏览: 17
以下是一个简单的Android Studio代码示例,包含登录页面和记事本备忘录功能:
```
// LoginActivity.java
public class LoginActivity extends AppCompatActivity {
private EditText usernameEditText;
private EditText passwordEditText;
private Button loginButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_login);
usernameEditText = findViewById(R.id.username_edit_text);
passwordEditText = findViewById(R.id.password_edit_text);
loginButton = findViewById(R.id.login_button);
loginButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String username = usernameEditText.getText().toString();
String password = passwordEditText.getText().toString();
// TODO: 在此处验证用户名和密码是否正确
Intent intent = new Intent(LoginActivity.this, MemoListActivity.class);
startActivity(intent);
}
});
}
}
```
```
// activity_login.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<EditText
android:id="@+id/username_edit_text"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="请输入用户名" />
<EditText
android:id="@+id/password_edit_text"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/username_edit_text"
android:hint="请输入密码"
android:inputType="textPassword" />
<Button
android:id="@+id/login_button"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/password_edit_text"
android:text="登录" />
</RelativeLayout>
```
```
// MemoListActivity.java
public class MemoListActivity extends AppCompatActivity {
private RecyclerView memoRecyclerView;
private MemoListAdapter memoListAdapter;
private List<Memo> memoList = new ArrayList<>();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_memo_list);
memoRecyclerView = findViewById(R.id.memo_recycler_view);
memoRecyclerView.setLayoutManager(new LinearLayoutManager(this));
memoListAdapter = new MemoListAdapter(memoList);
memoRecyclerView.setAdapter(memoListAdapter);
FloatingActionButton addMemoButton = findViewById(R.id.add_memo_button);
addMemoButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Intent intent = new Intent(MemoListActivity.this, MemoEditActivity.class);
startActivityForResult(intent, 1);
}
});
// TODO: 在此处从数据库中加载备忘录数据
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (requestCode == 1 && resultCode == RESULT_OK) {
Memo memo = new Memo(data.getStringExtra("title"), data.getStringExtra("body"));
memoList.add(memo);
memoListAdapter.notifyDataSetChanged();
// TODO: 在此处将新增的备忘录保存到数据库中
}
}
}
```
```
// activity_memo_list.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/memo_recycler_view"
android:layout_width="match_parent"
android:layout_height="match_parent" />
<com.google.android.material.floatingactionbutton.FloatingActionButton
android:id="@+id/add_memo_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:layout_alignParentEnd="true"
android:layout_margin="16dp"
android:src="@drawable/ic_add" />
</RelativeLayout>
```
```
// MemoEditActivity.java
public class MemoEditActivity extends AppCompatActivity {
private EditText memoTitleEditText;
private EditText memoBodyEditText;
private Button saveMemoButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_memo_edit);
memoTitleEditText = findViewById(R.id.memo_title_edit_text);
memoBodyEditText = findViewById(R.id.memo_body_edit_text);
saveMemoButton = findViewById(R.id.save_memo_button);
saveMemoButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent data = new Intent();
data.putExtra("title", memoTitleEditText.getText().toString());
data.putExtra("body", memoBodyEditText.getText().toString());
setResult(RESULT_OK, data);
finish();
}
});
}
}
```
```
// activity_memo_edit.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<EditText
android:id="@+id/memo_title_edit_text"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="请输入备忘录标题" />
<EditText
android:id="@+id/memo_body_edit_text"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/memo_title_edit_text"
android:hint="请输入备忘录内容"
android:inputType="textMultiLine"
android:minHeight="200dp" />
<Button
android:id="@+id/save_memo_button"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/memo_body_edit_text"
android:text="保存" />
</RelativeLayout>
```
上述代码实现了一个简单的登录页面和备忘录功能,其中:
- LoginActivity.java 实现了登录页面的逻辑,当用户输入用户名和密码后,点击登录按钮可以跳转到 MemoListActivity 页面。
- activity_login.xml 定义了登录页面的 UI 布局,包含用户名输入框、密码输入框和登录按钮。
- MemoListActivity.java 实现了备忘录列表的逻辑,包含备忘录列表的展示和新增备忘录的功能。
- activity_memo_list.xml 定义了备忘录列表页面的 UI 布局,包含一个 RecyclerView 和一个 FloatingActionButton。
- MemoEditActivity.java 实现了新增备忘录的逻辑,用户输入备忘录标题和内容后,点击保存按钮可以将备忘录保存到 MemoListActivity 页面的备忘录列表中。
- activity_memo_edit.xml 定义了新增备忘录页面的 UI 布局,包含备忘录标题输入框、备忘录内容输入框和保存按钮。
需要注意的是,上述代码仅仅是一个简单的示例,实际开发中还需要处理很多细节问题,例如用户登录状态的保存、备忘录数据的持久化、备忘录的编辑和删除等。
相关推荐
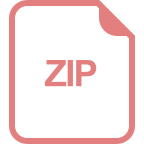














