python:将骑缝章图像放置在pdf上
时间: 2023-06-05 11:47:29 浏览: 70
在Python中,我们可以使用Pillow库来处理图像,PyPDF2库来处理PDF文件。首先,需要使用Pillow库将骑缝章图像加载进来,然后计算放置图像的位置。接着,使用PyPDF2库打开PDF文件,添加图像,并保存新的PDF文件。
具体步骤如下:
1. 安装需要的库
在命令行中输入以下命令来安装Pillow和PyPDF2库。
```
pip install Pillow
pip install PyPDF2
```
2. 加载图像
使用Pillow库的`Image.open()`函数加载骑缝章图像。
```python
from PIL import Image
image_path = 'path/to/image.jpg'
image = Image.open(image_path)
```
3. 计算放置位置
首先需要获取PDF页面的大小,可以通过PyPDF2库的`PdfFileReader()`和`getPage()`函数来获取。
```python
from PyPDF2 import PdfFileReader
pdf_path = 'path/to/file.pdf'
pdf_reader = PdfFileReader(open(pdf_path, 'rb'))
page = pdf_reader.getPage(0)
page_width = page.mediaBox.getWidth()
page_height = page.mediaBox.getHeight()
```
计算骑缝章图像的放置位置。这里假设骑缝章图像的大小是100x100像素,距离页面左边缘10像素,距离页面底边缘10像素。
```python
image_width = 100
image_height = 100
x = 10
y = 10
```
4. 添加图像
使用PyPDF2库的`PdfFileWriter()`和`addPage()`函数创建一个新的PDF文件,将原始PDF文件中的页面复制到新文件中,并在页面上添加骑缝章图像。
```python
from PyPDF2 import PdfFileReader, PdfFileWriter
from io import BytesIO
pdf_path = 'path/to/file.pdf'
pdf_reader = PdfFileReader(open(pdf_path, 'rb'))
pdf_writer = PdfFileWriter()
for page_num in range(pdf_reader.getNumPages()):
page = pdf_reader.getPage(page_num)
page_width = page.mediaBox.getWidth()
page_height = page.mediaBox.getHeight()
overlay = Image.new('RGB', (page_width, page_height), (255, 255, 255, 0))
overlay.paste(image, (x, page_height - y - image_height))
overlay_pdf = BytesIO()
overlay.save(overlay_pdf, format='png')
overlay_pdf.seek(0)
overlay_reader = PdfFileReader(overlay_pdf)
page.mergePage(overlay_reader.getPage(0))
pdf_writer.addPage(page)
with open('output.pdf', 'wb') as output_file:
pdf_writer.write(output_file)
```
最后,运行以上代码后,就会在`output.pdf`文件中看到添加了骑缝章图像的PDF文件了。
相关推荐
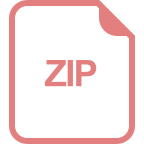
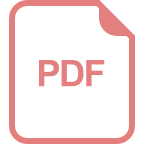













