逻辑回归Python
时间: 2023-11-29 21:45:22 浏览: 48
逻辑回归是一种二分类算法,它可以用于预测一个事件的发生概率。在Python中,我们可以使用原生Python实现逻辑回归算法。以下是一个简单的逻辑回归示例:
```python
import math
# 定义sigmoid函数
def sigmoid(x):
return 1 / (1 + math.exp(-x))
# 定义逻辑回归函数
def logistic_regression(features, weights):
# 计算线性组合
z = 0
for i in range(len(features)):
z += features[i] * weights[i]
# 计算sigmoid函数值
return sigmoid(z)
# 定义特征和权重
features = [1.2, 5.6, 3.4]
weights = [0.4, 1.2, -2.1]
# 计算预测概率
prediction = logistic_regression(features, weights)
# 输出预测概率
print("预测概率为:", prediction)
```
在上面的示例中,我们定义了sigmoid函数和逻辑回归函数。然后,我们定义了特征和权重,并使用逻辑回归函数计算预测概率。最后,我们输出了预测概率。
相关问题
逻辑回归python
在Python中实现逻辑回归可以使用多种库,比如Scikit-learn。下面是一个简单的逻辑回归示例:
首先导入所需库:
```python
import numpy as np
import pandas as pd
from sklearn.linear_model import LogisticRegression
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
```
读取数据集:
```python
data = pd.read_csv('data.csv')
```
使用train_test_split分割数据集:
```python
x_train, x_test, y_train, y_test = train_test_split(data.iloc[:, :-1], data.iloc[:, -1], test_size=0.2, random_state=42)
```
创建逻辑回归模型:
```python
lr = LogisticRegression()
```
训练模型:
```python
lr.fit(x_train, y_train)
```
预测测试集并计算准确率:
```python
y_pred = lr.predict(x_test)
accuracy = accuracy_score(y_test, y_pred)
print("Accuracy:", accuracy)
```
以上就是一个简单的逻辑回归示例。需要注意的是,逻辑回归适用于二分类问题,如果是多分类问题则需要使用其他算法或对逻辑回归进行扩展。
逻辑回归 python
逻辑回归是一种分类算法,它可以用于二元分类和多元分类问题。在 Python 中,可以使用 scikit-learn 库中的 LogisticRegression 类来实现逻辑回归。
首先,需要导入 LogisticRegression 类:
```python
from sklearn.linear_model import LogisticRegression
```
然后,可以使用 LogisticRegression 类来拟合数据并进行预测。以下是一个简单的例子:
```python
import numpy as np
from sklearn.linear_model import LogisticRegression
# 生成一些随机数据
X = np.random.randn(100, 2)
y = (X[:, 0] + X[:, 1] > 0).astype(int)
# 创建逻辑回归模型
model = LogisticRegression()
# 拟合数据
model.fit(X, y)
# 进行预测
y_pred = model.predict(X)
# 输出预测结果
print(y_pred)
```
在这个例子中,首先生成了一些随机数据,然后使用 LogisticRegression 类创建了一个逻辑回归模型。接着,使用 fit() 方法拟合数据,并使用 predict() 方法进行预测。最后,使用 print() 函数输出预测结果。
需要注意的是,逻辑回归模型需要对特征进行标准化处理,以确保最好的性能。可以使用 scikit-learn 中的 StandardScaler 类来标准化特征。
相关推荐
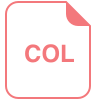
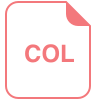
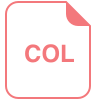
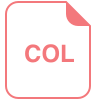
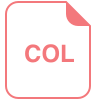






