使用Kestrel创建tcp服务器
时间: 2024-03-20 14:40:17 浏览: 219
要使用Kestrel创建TCP服务器,您需要使用`Microsoft.AspNetCore.Server.Kestrel.Transport.Sockets` NuGet包。以下是一个示例,演示如何使用Kestrel创建TCP服务器:
```csharp
using System;
using System.Net;
using System.Net.Sockets;
using System.Threading.Tasks;
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Hosting;
using Microsoft.AspNetCore.Server.Kestrel.Transport.Sockets;
class Program
{
static async Task Main(string[] args)
{
var host = new WebHostBuilder()
.UseKestrel(options =>
{
options.Listen(IPAddress.Any, 1234, listenOptions =>
{
listenOptions.UseConnectionHandler<TcpConnectionHandler>();
});
})
.ConfigureServices(services => services.AddSingleton<TcpConnectionHandler>())
.Configure(app => app.UseRouting())
.Build();
await host.RunAsync();
}
}
class TcpConnectionHandler : ConnectionHandler
{
public override async Task OnConnectedAsync(ConnectionContext connection)
{
Console.WriteLine($"New client connected: {connection.ConnectionId}");
while (true)
{
var buffer = new byte[1024];
var result = await connection.Transport.Input.ReadAsync(buffer);
var input = Encoding.UTF8.GetString(buffer.Slice(0, result.Buffer.Length));
Console.WriteLine($"Received from client {connection.ConnectionId}: {input}");
if (result.IsCompleted)
{
break;
}
}
}
}
```
在此示例中,`Listen`方法用于配置Kestrel服务器以侦听TCP连接。使用`UseConnectionHandler`方法指定连接处理程序,该处理程序将处理所有传入的连接。在此示例中,我们使用`TcpConnectionHandler`类作为连接处理程序。在`TcpConnectionHandler`的`OnConnectedAsync`方法中,我们读取来自客户端的数据并打印到控制台上。
阅读全文
相关推荐
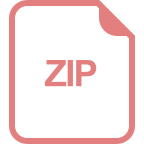
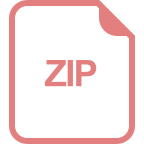
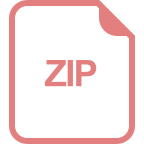

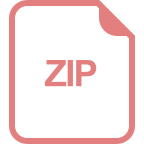
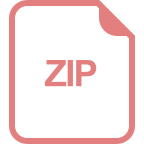
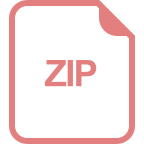
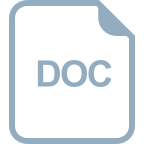


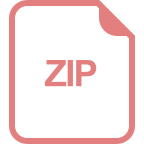
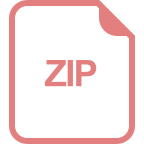
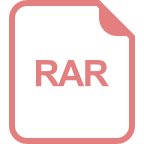
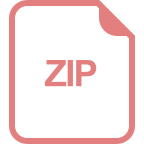
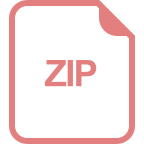
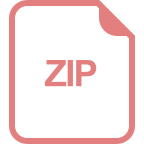