用vue3和node写博客评论接口
时间: 2024-05-12 11:19:01 浏览: 99
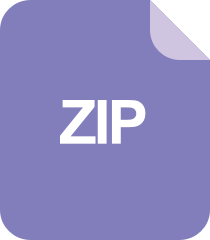
使用vue和node开发的博客系统
以下是一个简单的示例,使用Vue 3和Node.js编写博客评论API。
1.创建Vue 3应用程序
首先,我们需要创建一个Vue 3应用程序,以便用户可以在浏览器中发表评论。我们将使用Vue CLI来创建应用程序:
```
vue create blog-comments
```
在创建应用程序时,您可以选择使用默认设置或自定义设置。对于此示例,使用默认设置即可。
2.创建Node.js服务器
接下来,我们需要创建一个Node.js服务器来处理评论。我们将使用Express框架来处理HTTP请求和响应。
在项目文件夹中,使用以下命令来安装Express:
```
npm install express
```
然后,创建一个名为server.js的文件并将以下代码添加到其中:
```javascript
const express = require('express');
const app = express();
const bodyParser = require('body-parser');
const cors = require('cors');
app.use(bodyParser.json());
app.use(cors());
const comments = [];
app.get('/comments', (req, res) => {
res.send(comments);
});
app.post('/comments', (req, res) => {
const { name, comment } = req.body;
comments.push({ name, comment });
res.send('Comment added.');
});
app.listen(4000, () => {
console.log('Server is running on port 4000.');
});
```
在这里,我们创建了一个Express应用程序,使用body-parser中间件解析请求正文,使用CORS中间件允许跨域请求,并创建了一个comments数组来保存所有评论。
我们还定义了两个路由:一个用于获取所有评论,另一个用于添加新评论。在添加新评论时,我们从请求正文中获取评论的名称和内容,并将其添加到comments数组中。
最后,我们将服务器运行在端口4000上。
3.将Vue应用程序连接到Node.js服务器
现在,我们需要将Vue应用程序连接到Node.js服务器,使用户能够发表评论并查看所有评论。
打开src/main.js文件并将以下代码添加到其中:
```javascript
import { createApp } from 'vue';
import App from './App.vue';
const app = createApp(App);
app.config.globalProperties.api = 'http://localhost:4000';
app.mount('#app');
```
在这里,我们使用Vue 3的createApp函数创建了Vue应用程序,并将服务器的URL存储在全局属性api中。
接下来,在src/App.vue文件中,我们可以使用以下代码来获取和添加评论:
```javascript
<script>
export default {
data() {
return {
name: '',
comment: '',
comments: []
};
},
created() {
this.getComments();
},
methods: {
async getComments() {
const response = await fetch(`${this.$api}/comments`);
this.comments = await response.json();
},
async addComment() {
const response = await fetch(`${this.$api}/comments`, {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({ name: this.name, comment: this.comment })
});
this.name = '';
this.comment = '';
this.getComments();
}
}
};
</script>
<template>
<div>
<h1>Blog Comments</h1>
<form @submit.prevent="addComment">
<label>
Name:
<input v-model="name" type="text" required />
</label>
<label>
Comment:
<textarea v-model="comment" required></textarea>
</label>
<button type="submit">Submit</button>
</form>
<div v-for="comment in comments" :key="comment.comment">
<h3>{{ comment.name }}</h3>
<p>{{ comment.comment }}</p>
</div>
</div>
</template>
```
在这里,我们使用Vue的fetch方法从服务器获取评论,并使用相同的方法将新评论添加到服务器。我们还在组件上使用v-model指令来绑定输入字段和数据属性。
最后,我们在模板中使用v-for指令来遍历所有评论,并使用{{}}插值语法在页面上呈现它们。
现在,您可以启动Vue应用程序和Node.js服务器,并在浏览器中访问应用程序以测试评论功能。
阅读全文
相关推荐
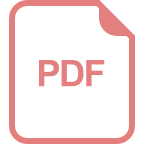
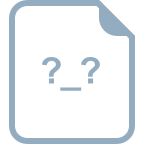
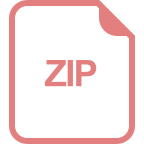
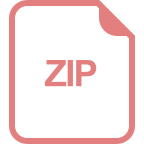
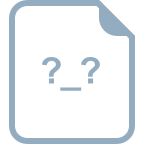
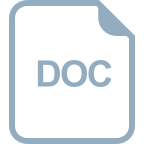
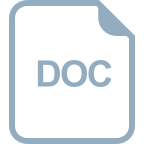
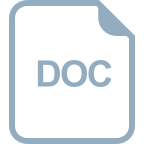
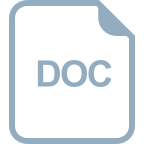
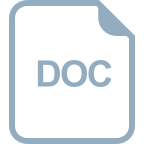
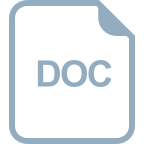
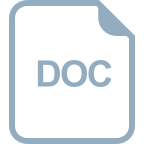
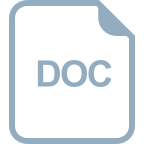




