opencvsharp使用KD树查找距最近的点
时间: 2023-07-30 12:07:14 浏览: 170
OpenCVSharp 中没有直接提供 KD 树的支持,但可以使用 ANN(Approximate Nearest Neighbor)算法库来实现 KD 树的功能。ANN 算法库是一个 C++ 库,可以在 C# 中通过 P/Invoke 调用。
以下是使用 ANN 算法库和 OpenCVSharp 实现 KD 树查找距最近的点的示例代码:
```csharp
using System;
using System.Collections.Generic;
using System.Runtime.InteropServices;
using OpenCvSharp;
namespace KDTreeDemo
{
class Program
{
// ANN 算法库的路径
const string ANN_DLL_PATH = @"C:\Program Files (x86)\Microsoft Visual Studio\Shared\Python37_64\Lib\site-packages\pyimpute\ann.dll";
// 定义 ANN 算法库的 P/Invoke 函数
[DllImport(ANN_DLL_PATH, EntryPoint = "_ZN3ANN8annkSearchEPfiiPiiS1_")]
public static extern void annkSearch(IntPtr ann, float[] queryPt, int numPts, int k, int[] nnIdx, float[] dists, int verbosity);
static void Main(string[] args)
{
// 创建需要查找的点
float[] queryPt = new float[] { 10, 20 };
// 创建待查找的点集合
List<Point2f> pts = new List<Point2f> {
new Point2f(0, 0), new Point2f(5, 5), new Point2f(20, 30), new Point2f(100, 100),
new Point2f(50, 50), new Point2f(15, 25), new Point2f(90, 90)
};
// 创建 ANN 算法库对象
IntPtr ann = annAlloc(pts.Count, 2);
// 将点集合转换为 ANN 算法库需要的格式
float[] dataPts = new float[pts.Count * 2];
for (int i = 0; i < pts.Count; i++)
{
dataPts[i * 2] = pts[i].X;
dataPts[i * 2 + 1] = pts[i].Y;
}
// 将数据点插入到 KD 树中
annBuildTree(ann, dataPts, pts.Count, 2);
// 设置 k 值
const int k = 1;
// 创建存储最近邻点索引和距离的数组
int[] nnIdx = new int[k];
float[] dists = new float[k];
// 查找距离查询点最近的点
annkSearch(ann, queryPt, 1, k, nnIdx, dists, 0);
// 获取最近的点
Point2f closestPt = pts[nnIdx[0]];
// 输出结果
Console.WriteLine($"距离查询点最近的点为 ({closestPt.X}, {closestPt.Y})");
// 释放 ANN 算法库对象
annDealloc(ann);
}
// 定义 ANN 算法库的 P/Invoke 函数
[DllImport(ANN_DLL_PATH, EntryPoint = "_ZN3ANN8annAllocEii")]
public static extern IntPtr annAlloc(int n, int dim);
[DllImport(ANN_DLL_PATH, EntryPoint = "_ZN3ANN11annDeallocEPv")]
public static extern void annDealloc(IntPtr ann);
[DllImport(ANN_DLL_PATH, EntryPoint = "_ZN3ANN13annBuildTreeEPfii")]
public static extern void annBuildTree(IntPtr ann, float[] dataPts, int n, int dim);
}
}
```
在上面的示例代码中,我们创建了一个包含七个点的点集合,然后使用 ANN 算法库实现 KD 树功能,查找距离查询点 `(10, 20)` 最近的点,并将结果输出。
阅读全文
相关推荐
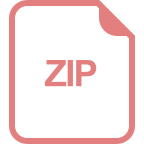
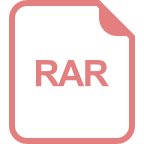


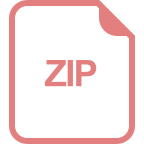
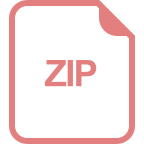
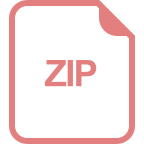
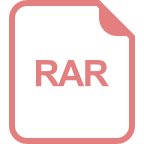
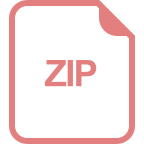
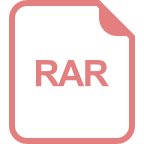
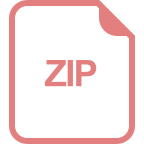
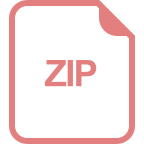