scala 决策树 淘宝回头客 混交矩阵 roc曲线 代码
时间: 2023-10-11 17:19:43 浏览: 51
以下是使用Scala实现决策树算法、混淆矩阵和ROC曲线的代码,用于预测淘宝回头客:
1. 决策树算法
```scala
import org.apache.spark.ml.classification.DecisionTreeClassifier
import org.apache.spark.ml.feature.VectorAssembler
// 加载数据
val data = spark.read.format("csv").option("header", true).load("file_path")
// 将数据转换为二元分类问题
val binaryData = data.withColumn("label", when(col("回头客") === "是", 1.0).otherwise(0.0))
val featureCols = binaryData.columns.filter(_ != "回头客")
// 将特征向量转换为向量列
val assembler = new VectorAssembler().setInputCols(featureCols).setOutputCol("features")
val binaryDataWithFeatures = assembler.transform(binaryData)
// 拆分数据集为训练集和测试集
val Array(trainingData, testData) = binaryDataWithFeatures.randomSplit(Array(0.7, 0.3), seed = 1234L)
// 训练决策树模型
val model = new DecisionTreeClassifier().setLabelCol("label").setFeaturesCol("features").fit(trainingData)
// 测试模型
val predictions = model.transform(testData)
// 输出预测结果
predictions.show()
```
2. 混淆矩阵
```scala
import org.apache.spark.mllib.evaluation.MulticlassMetrics
// 将预测结果转换为RDD
val predictionAndLabels = predictions.select("prediction", "label")
.rdd.map(row => (row.getDouble(0), row.getDouble(1)))
// 计算混淆矩阵
val metrics = new MulticlassMetrics(predictionAndLabels)
val confusionMatrix = metrics.confusionMatrix
// 输出混淆矩阵
println(s"Confusion matrix:\n$confusionMatrix")
```
3. ROC曲线
```scala
import org.apache.spark.mllib.evaluation.BinaryClassificationMetrics
// 计算预测得分
val scoreAndLabels = predictions.select("probability", "label")
.rdd.map(row => (row.getAs[org.apache.spark.ml.linalg.Vector](0)(1), row.getDouble(1)))
// 计算ROC曲线
val metrics = new BinaryClassificationMetrics(scoreAndLabels)
val roc = metrics.roc
// 输出ROC曲线
println(s"ROC curve:\n$roc")
```
请注意,这些代码是为了演示用途而编写的,并且可能需要根据您的数据和任务进行修改和优化。
相关推荐
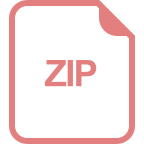
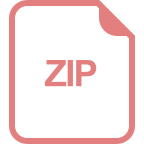














