用python构建随机点TIN和加权V图
时间: 2023-06-18 11:08:57 浏览: 67
构建随机点TIN和加权V图可以使用Python中的Delaunay三角剖分算法和Voronoi图算法。下面是一个简单的例子:
首先,我们需要生成随机点。可以使用Python的NumPy库生成随机坐标:
```python
import numpy as np
num_points = 20
points = np.random.rand(num_points, 2) # 生成20个随机点
```
接下来,我们使用SciPy库中的Delaunay函数进行三角剖分:
```python
from scipy.spatial import Delaunay
tri = Delaunay(points)
```
现在,我们可以使用Matplotlib库将三角形可视化:
```python
import matplotlib.pyplot as plt
plt.triplot(points[:,0], points[:,1], tri.simplices)
plt.plot(points[:,0], points[:,1], 'o')
plt.show()
```
如果要生成加权V图,可以在生成随机点时为每个点分配一个权重。然后,在进行Delaunay三角剖分之前,可以根据权重对点进行排序:
```python
weights = np.random.rand(num_points)
sorted_points = points[np.argsort(weights)]
tri = Delaunay(sorted_points)
```
最后,可以使用Scipy库中的Voronoi函数生成Voronoi图:
```python
from scipy.spatial import Voronoi
vor = Voronoi(sorted_points)
```
现在,我们可以使用Matplotlib库将Voronoi图可视化:
```python
plt.plot(sorted_points[:,0], sorted_points[:,1], 'o')
plt.plot(vor.vertices[:,0], vor.vertices[:,1], 'rx')
for simplex in vor.ridge_vertices:
if np.all(simplex >= 0):
plt.plot(vor.vertices[simplex,0], vor.vertices[simplex,1], 'k-')
plt.show()
```
这样就可以生成随机点的TIN和加权V图了。
相关推荐
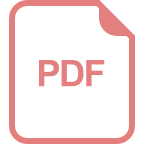
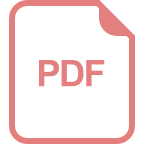
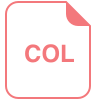
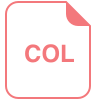
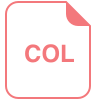
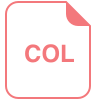
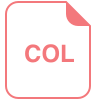









