Java TTLCache怎么写
时间: 2023-08-31 10:31:49 浏览: 96
### 回答1:
Java TTLCache 是一个可以自动过期的缓存工具类,可以用于保存一些临时数据,数据在指定的时间后自动从缓存中删除,以避免占用过多的内存。
下面是一个简单的实现示例:
```java
import java.util.Map;
import java.util.HashMap;
public class TTLCache<K, V> {
private Map<K, CacheEntry<V>> cache = new HashMap<>();
public synchronized void put(K key, V value, long ttl) {
long expiryTime = System.currentTimeMillis() + ttl;
CacheEntry<V> entry = new CacheEntry<>(value, expiryTime);
cache.put(key, entry);
}
public synchronized V get(K key) {
CacheEntry<V> entry = cache.get(key);
if (entry == null) {
return null;
}
if (entry.expired()) {
cache.remove(key);
return null;
}
return entry.value;
}
private static class CacheEntry<V> {
private V value;
private long expiryTime;
public CacheEntry(V value, long expiryTime) {
this.value = value;
this.expiryTime = expiryTime;
}
public boolean expired() {
return System.currentTimeMillis() >= expiryTime;
}
}
}
```
这个类使用了一个 HashMap 来保存缓存数据,当数据过期时,会自动从 HashMap 中删除。put 方法用来向缓存中添加数据,ttl 参数表示数据的过期时间(以毫秒为单位)。get 方法用来获取缓存中的数据,如果数据过期或者不存在,返回 null。为了保证线程安全,这个类使用了 synchronized 关键字。
这只是一个简单的实现示例,实际使用中可能需要添加更多的功能,比如清空缓存、设置默认的过期时间等。
### 回答2:
Java TTLCache是一种具有过期时间的缓存实现。下面是一个简单的实现示例:
首先,我们需要一个存储键值对的容器来表示缓存。可以使用HashMap来保存键值对,并且在每个值上存储一个表示过期时间的时间戳。
```java
import java.util.HashMap;
public class TTLCache<K, V> {
private HashMap<K, CacheObject> cache;
public TTLCache() {
cache = new HashMap<>();
}
public synchronized void put(K key, V value, long ttl) {
long expirationTime = System.currentTimeMillis() + ttl;
cache.put(key, new CacheObject(value, expirationTime));
}
public synchronized V get(K key) {
CacheObject obj = cache.get(key);
if (obj != null && obj.expirationTime >= System.currentTimeMillis()) {
return obj.value;
}
return null;
}
private class CacheObject {
private V value;
private long expirationTime;
public CacheObject(V value, long expirationTime) {
this.value = value;
this.expirationTime = expirationTime;
}
}
}
```
在上面的示例中,我们定义了一个TTLCache类,使用HashMap作为内部存储。构造函数初始化了缓存容器。
put方法用于向缓存中放入一个键值对,并指定过期时间。它首先计算出过期时间戳,然后将键和包含值和过期时间的CacheObject对象存储到HashMap中。
get方法用于从缓存中获取一个值。它首先检查键是否存在于缓存中,并且检查该值的过期时间是否大于当前时间。如果是,那么返回对应的值,否则返回null。
注意,这里的put和get方法都使用了synchronized关键字来确保线程安全。
这只是一个简单的TTLCache示例,你可以根据自己的需求进行扩展和改进。例如,可以实现自动清理过期键值对的功能,以及引入LRU缓存策略等。
### 回答3:
Java TTLCache是一个带有时间限制的缓存实现,它会在一段特定的时间内存储数据,并在超过该时间后自动删除数据。下面是一个简单的Java TTLCache的实现例子:
```java
import java.util.HashMap;
import java.util.Map;
public class TTLCache<K, V> {
private final long timeToLive; // 缓存数据的时间限制
private final Map<K, CacheObject<V>> cacheMap; // 用于存储缓存数据的Map
// 定义缓存数据对象,保存数据和数据创建时间
private static class CacheObject<T> {
private final T data;
private final long createTime;
public CacheObject(T data) {
this.data = data;
this.createTime = System.currentTimeMillis();
}
}
public TTLCache(long timeToLive) {
this.timeToLive = timeToLive;
this.cacheMap = new HashMap<>();
}
public synchronized void put(K key, V value) {
cacheMap.put(key, new CacheObject<>(value));
}
public synchronized V get(K key) {
CacheObject<V> cacheObject = cacheMap.get(key);
if (cacheObject == null) {
return null;
}
long currentTime = System.currentTimeMillis();
// 如果数据超过时间限制,则移除数据并返回null
if (currentTime - cacheObject.createTime > timeToLive) {
cacheMap.remove(key);
return null;
}
return cacheObject.data;
}
public synchronized void remove(K key) {
cacheMap.remove(key);
}
public synchronized void clear() {
cacheMap.clear();
}
public synchronized int size() {
return cacheMap.size();
}
}
```
上述实现中,我们使用了一个HashMap来存储缓存数据,并使用泛型来支持不同类型的数据。在CacheObject类中,我们保存了数据的创建时间,然后在get方法中判断数据是否超过了时间限制,如果超过则移除数据并返回null。最后我们提供了一些基本的方法,如添加数据的put方法,获取数据的get方法,移除指定数据的remove方法,清空所有数据的clear方法以及获取缓存大小的size方法。
使用这个TTLCache,我们可以简单地使用如下代码进行缓存操作:
```java
TTLCache<String, Integer> cache = new TTLCache<>(1000); // 设置缓存时间为1秒
cache.put("key1", 10);
cache.put("key2", 20);
Integer value1 = cache.get("key1"); // 获取数据,返回10
Integer value2 = cache.get("key2"); // 获取数据,返回20
Thread.sleep(2000); // 等待2秒,超过缓存时间限制
Integer value3 = cache.get("key1"); // 获取数据,返回null,因为数据已经过期
```
通过使用Java TTLCache,我们可以实现一个简单的带有时间限制的缓存,并根据业务需求自定义缓存的时间限制。
相关推荐
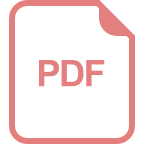
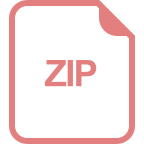
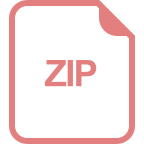














