hashmap实现wordcount
时间: 2023-09-02 20:15:37 浏览: 93
可以使用Java中的HashMap来实现wordcount功能。具体实现方法如下:
1. 定义一个HashMap,用于存储单词及其出现的次数。
2. 将文本内容分割成单词,可以使用Java中的String的split方法或者使用正则表达式进行分割。
3. 遍历分割后的单词数组,将每个单词作为HashMap的key,如果该单词已经在HashMap中存在,则将其对应的value加1,否则将其加入HashMap,并将其value设置为1。
4. 遍历完单词数组后,HashMap中存储的就是每个单词及其出现的次数,可以遍历HashMap,输出每个单词及其出现的次数。
以下是示例代码:
```
import java.util.*;
public class WordCount {
public static void main(String[] args) {
String text = "This is a test. This is only a test.";
Map<String, Integer> wordCountMap = new HashMap<String, Integer>();
String[] words = text.split("\\W+"); // 使用正则表达式分割单词
for (String word : words) {
if (wordCountMap.containsKey(word)) {
// 如果单词已存在,则将其value加1
wordCountMap.put(word, wordCountMap.get(word) + 1);
} else {
// 如果单词不存在,则将其加入HashMap,并将其value设置为1
wordCountMap.put(word, 1);
}
}
// 遍历HashMap,输出每个单词及其出现的次数
for (String word : wordCountMap.keySet()) {
System.out.println(word + ": " + wordCountMap.get(word));
}
}
}
```
输出结果为:
```
This: 2
a: 2
test: 2
only: 1
```
阅读全文
相关推荐
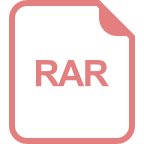
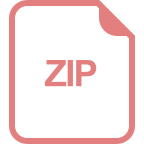
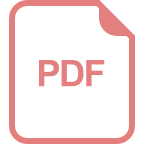
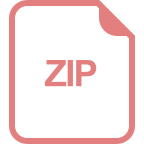
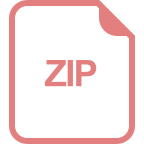
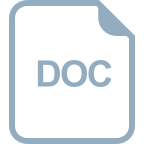
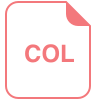
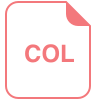
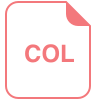
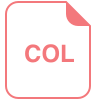








