请使用java写一个写一个二叉树
时间: 2023-08-31 17:43:28 浏览: 110
### 回答1:
可以使用以下代码实现二叉树:
```
class Node {
int data;
Node left;
Node right;
public Node(int data) {
this.data = data;
this.left = null;
this.right = null;
}
}
class BinaryTree {
Node root;
public BinaryTree() {
root = null;
}
public void addNode(int data) {
root = addNodeRecursive(root, data);
}
private Node addNodeRecursive(Node current, int data) {
if (current == null) {
return new Node(data);
}
if (data < current.data) {
current.left = addNodeRecursive(current.left, data);
} else if (data > current.data) {
current.right = addNodeRecursive(current.right, data);
} else {
return current;
}
return current;
}
}
```
这是一个简单的二叉树实现。您可以使用`addNode`方法向树中添加节点,并递归调用`addNodeRecursive`方法来确定节点的位置。
### 回答2:
二叉树是一种常见的数据结构,可以通过Java编程语言来实现。
首先,我们需要定义二叉树的节点类。节点类包含两个属性:左子节点和右子节点,以及一个用于存储数据的变量。代码如下:
```java
class TreeNode {
int data;
TreeNode left;
TreeNode right;
public TreeNode(int data) {
this.data = data;
this.left = null;
this.right = null;
}
}
```
接下来,我们可以创建一个二叉树类,包含插入节点和遍历二叉树的方法。代码如下:
```java
class BinaryTree {
TreeNode root;
public BinaryTree() {
root = null;
}
public void insert(int data) {
root = insertHelper(root, data);
}
private TreeNode insertHelper(TreeNode current, int data) {
if (current == null) {
return new TreeNode(data);
}
if (data < current.data) {
current.left = insertHelper(current.left, data);
} else if (data > current.data) {
current.right = insertHelper(current.right, data);
}
return current;
}
public void inorderTraversal() {
inorderTraversalHelper(root);
}
private void inorderTraversalHelper(TreeNode current) {
if (current != null) {
inorderTraversalHelper(current.left);
System.out.print(current.data + " ");
inorderTraversalHelper(current.right);
}
}
}
```
最后,我们可以在主函数中创建一个二叉树对象,并插入一些节点,并对二叉树进行中序遍历。代码如下:
```java
public class Main {
public static void main(String[] args) {
BinaryTree binaryTree = new BinaryTree();
binaryTree.insert(5);
binaryTree.insert(3);
binaryTree.insert(7);
binaryTree.insert(1);
binaryTree.insert(4);
binaryTree.insert(6);
binaryTree.insert(8);
System.out.println("中序遍历结果:");
binaryTree.inorderTraversal();
}
}
```
以上代码中,我们首先创建了一个空的二叉树对象。然后,通过插入方法向二叉树中插入节点,节点的值可以根据具体需求进行修改。最后,我们调用中序遍历方法,输出二叉树的节点值。
这样,我们就实现了一个简单的二叉树。当然,二叉树还有其他常见的操作,如前序遍历、后序遍历、删除节点等,可以根据实际需求进行扩展。
### 回答3:
使用Java编写一个二叉树的实现代码如下:
```java
class Node {
int value;
Node left;
Node right;
public Node(int value) {
this.value = value;
this.left = null;
this.right = null;
}
}
class BinaryTree {
Node root;
public BinaryTree() {
this.root = null;
}
public void insert(int value) {
this.root = insertNode(this.root, value);
}
private Node insertNode(Node root, int value) {
if (root == null) {
root = new Node(value);
return root;
}
if (value < root.value) {
root.left = insertNode(root.left, value);
} else if (value > root.value) {
root.right = insertNode(root.right, value);
}
return root;
}
public void inorderTraversal() {
inorderTraversal(this.root);
}
private void inorderTraversal(Node root) {
if (root != null) {
inorderTraversal(root.left);
System.out.print(root.value + " ");
inorderTraversal(root.right);
}
}
}
public class Main {
public static void main(String[] args) {
BinaryTree tree = new BinaryTree();
// 插入节点示例
tree.insert(10);
tree.insert(5);
tree.insert(15);
tree.insert(3);
tree.insert(8);
tree.insert(12);
tree.insert(18);
// 中序遍历示例
tree.inorderTraversal(); // 输出:3 5 8 10 12 15 18
}
}
```
此代码实现了一个简单的二叉树,并包含了插入节点和中序遍历的功能。使用`Node`类表示每个节点,包含节点值、左子节点和右子节点。`BinaryTree`类作为二叉树的入口,包含根节点以及插入节点和中序遍历的方法。在`Main`类中,我们创建一个二叉树对象,通过调用插入方法向树中插入节点,并通过调用中序遍历方法打印出节点值。
阅读全文
相关推荐
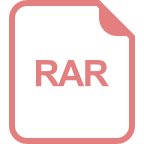
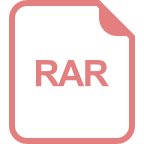
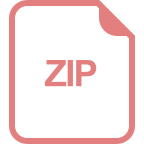

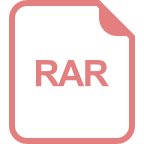
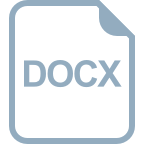
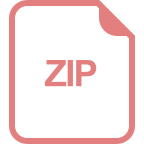
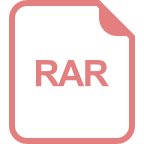
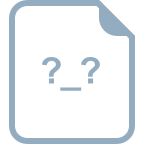
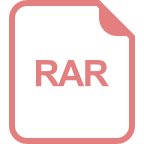
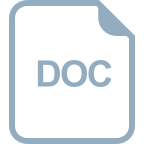