python截取字符串后面一半
时间: 2023-10-14 15:26:40 浏览: 230
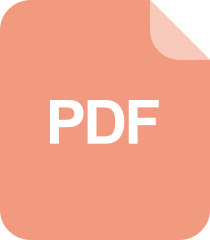
python 截取 取出一部分的字符串方法
可以使用字符串切片来截取字符串的后半部分。假设字符串为s,可以使用以下代码:
```
s = "abcdefg"
half_len = len(s) // 2
second_half = s[half_len:]
print(second_half)
```
输出结果为:
```
defg
```
这里先计算了字符串长度的一半,然后使用切片取出从一半位置到字符串结尾的部分。
阅读全文
相关推荐
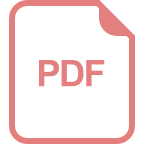
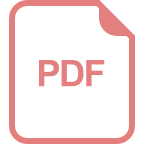
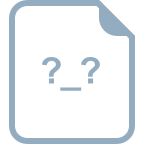
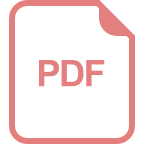
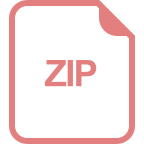
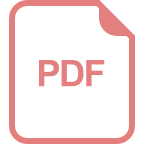
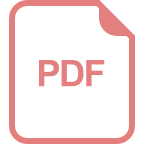
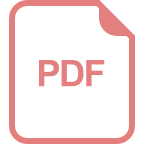
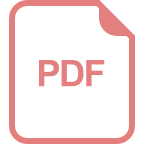
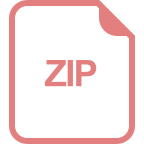
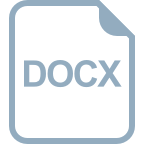
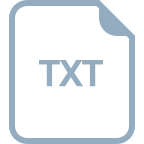
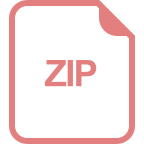