C语言自动识别网页类型
时间: 2023-07-11 09:07:08 浏览: 105
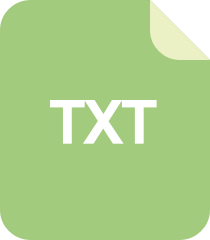
C语言读网页

在C语言中,可以通过使用libcurl库来请求网页,然后解析返回的HTTP响应头中的Content-Type字段来自动识别网页类型。
具体步骤如下:
1. 安装libcurl库并包含相关头文件和链接库。
2. 使用curl_easy_init()函数初始化一个curl句柄。
3. 使用curl_easy_setopt()函数设置curl选项,如设置请求的URL、设置是否跟踪重定向等。
4. 使用curl_easy_perform()函数执行请求,获取返回的HTTP响应头。
5. 解析HTTP响应头中的Content-Type字段,判断网页类型。
以下是一个简单的示例代码:
```
#include <stdio.h>
#include <curl/curl.h>
int main(void)
{
CURL *curl;
CURLcode res;
curl_global_init(CURL_GLOBAL_DEFAULT);
curl = curl_easy_init();
if (curl) {
curl_easy_setopt(curl, CURLOPT_URL, "https://www.baidu.com");
res = curl_easy_perform(curl);
if (res == CURLE_OK) {
char *content_type;
res = curl_easy_getinfo(curl, CURLINFO_CONTENT_TYPE, &content_type);
if (res == CURLE_OK && content_type) {
if (strstr(content_type, "text/html")) {
printf("This is a HTML page.\n");
} else if (strstr(content_type, "application/json")) {
printf("This is a JSON page.\n");
} else {
printf("Unknown page type.\n");
}
}
}
curl_easy_cleanup(curl);
}
curl_global_cleanup();
return 0;
}
```
该示例代码请求百度首页并判断其网页类型。如果Content-Type字段中包含"text/html"字符串,则认为是HTML页面;如果包含"application/json"字符串,则认为是JSON数据;否则认为是未知类型。
阅读全文
相关推荐
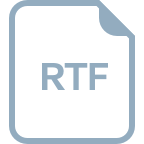
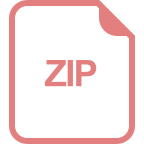

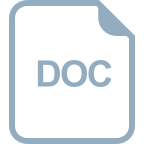
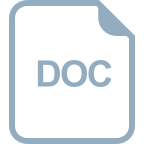
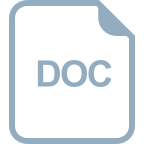
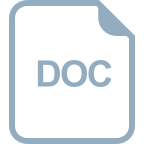
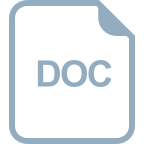
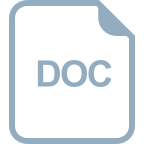
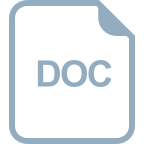
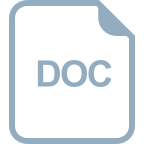
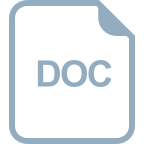
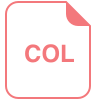
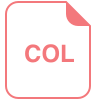
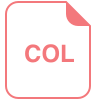
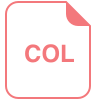
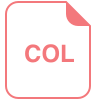