ubuntu环境下使用c语言实现车牌检测
时间: 2023-10-07 19:14:13 浏览: 173
要在Ubuntu环境下使用C语言实现车牌检测,可以按照以下步骤:
1. 安装OpenCV库:在终端中输入以下命令安装OpenCV库:
```
sudo apt-get install libopencv-dev
```
2. 编写C语言代码:使用OpenCV库提供的函数,例如cvLoadImage、cvCvtColor、cvSmooth、cvThreshold等,编写车牌检测的C语言代码。
3. 编译代码:在终端中使用以下命令编译代码:
```
gcc -o program program.c `pkg-config --cflags --libs opencv`
```
其中,program.c为你编写的C语言代码的文件名,program为编译后生成的可执行文件名。
4. 运行程序:在终端中使用以下命令运行程序:
```
./program
```
注意,以上步骤仅为概述,具体的实现细节需要根据具体的车牌检测算法进行调整。
阅读全文
相关推荐
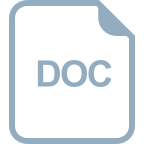
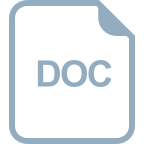
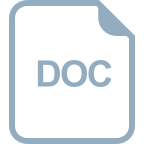
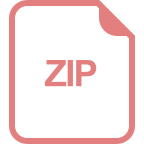
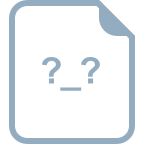
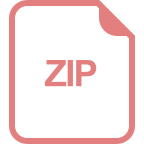
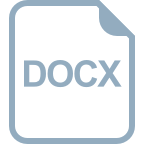
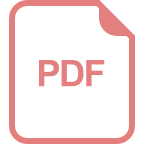
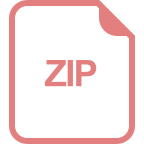
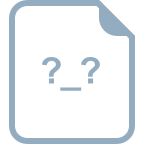
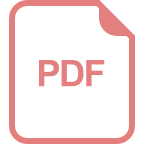
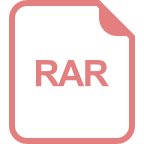
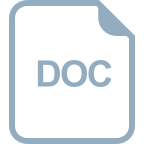
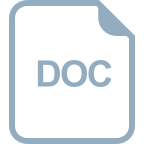
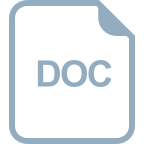
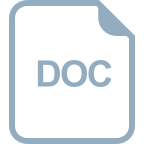