java实现商品信息管理系统代码
时间: 2024-06-23 19:00:56 浏览: 228
Java 实现一个商品信息管理系统通常会涉及到多个模块,包括数据库连接、用户管理、商品添加编辑、查询和删除等。这里我会给出一个简化的概述,但请注意,这只是一个概念性的框架,实际的代码实现会更复杂,并且需要使用具体的库(如JDBC、Spring Data JPA等)。
1. 数据模型 (Entities) - 商品类 (Product):
```java
public class Product {
@Id
private Long id;
private String name;
private String description;
private double price;
// getters and setters
}
```
2. 数据访问层 (Repository) - 商品仓库 (ProductRepository):
```java
import org.springframework.data.jpa.repository.JpaRepository;
public interface ProductRepository extends JpaRepository<Product, Long> {
List<Product> findAll();
// 添加/更新/删除方法
}
```
3. 服务层 (Service) - 商品服务 (ProductService):
```java
import org.springframework.beans.factory.annotation.Autowired;
public class ProductService {
private final ProductRepository productRepository;
@Autowired
public ProductService(ProductRepository productRepository) {
this.productRepository = productRepository;
}
public void save(Product product) {
productRepository.save(product);
}
// 其他业务方法,如查找、修改和删除
}
```
4. 控制器层 (Controller) - 前端接口处理:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
@RestController
@RequestMapping("/api/products")
public class ProductController {
@Autowired
private ProductService productService;
@PostMapping
public ResponseEntity<?> create(@RequestBody Product product) {
productService.save(product);
return ResponseEntity.ok().build();
}
// 用类似的方法处理GET, PUT, DELETE请求
}
```
阅读全文
相关推荐
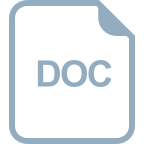
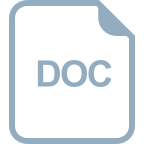
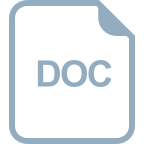
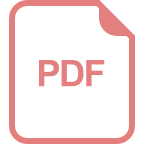
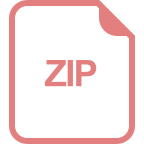
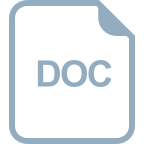
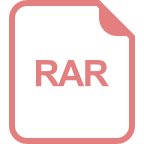
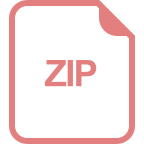
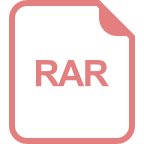
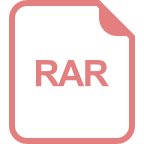
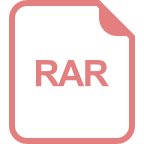
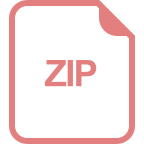
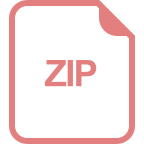
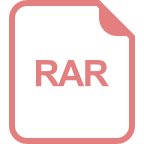
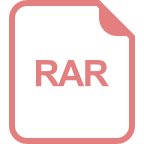
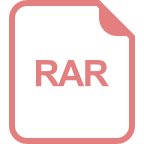
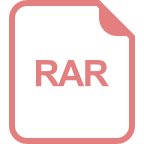
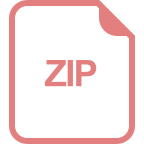
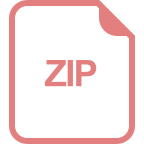