android studio 用listview和fragment创建购物商城,商城底部有首页,商品信息,购物车和个人中心四个按钮,点击按钮会实现fragment转换页面的代码
时间: 2024-05-01 15:21:51 浏览: 215
好的,以下是你需要的代码:
首先,在你的布局文件中添加底部导航栏,例如:
```
<LinearLayout
android:id="@+id/bottom_navigation"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:background="#ffffff"
android:elevation="8dp"
android:layout_alignParentBottom="true">
<ImageButton
android:id="@+id/home_button"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:src="@drawable/ic_home"
android:background="?attr/selectableItemBackgroundBorderless"/>
<ImageButton
android:id="@+id/products_button"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:src="@drawable/ic_products"
android:background="?attr/selectableItemBackgroundBorderless"/>
<ImageButton
android:id="@+id/cart_button"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:src="@drawable/ic_cart"
android:background="?attr/selectableItemBackgroundBorderless"/>
<ImageButton
android:id="@+id/profile_button"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:src="@drawable/ic_profile"
android:background="?attr/selectableItemBackgroundBorderless"/>
</LinearLayout>
```
然后,在你的Activity中实现底部导航栏的点击事件和Fragment的切换逻辑,例如:
```
public class MainActivity extends AppCompatActivity {
private Fragment homeFragment;
private Fragment productsFragment;
private Fragment cartFragment;
private Fragment profileFragment;
private Fragment activeFragment;
private ImageButton homeButton;
private ImageButton productsButton;
private ImageButton cartButton;
private ImageButton profileButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
homeFragment = new HomeFragment();
productsFragment = new ProductsFragment();
cartFragment = new CartFragment();
profileFragment = new ProfileFragment();
activeFragment = homeFragment;
homeButton = findViewById(R.id.home_button);
productsButton = findViewById(R.id.products_button);
cartButton = findViewById(R.id.cart_button);
profileButton = findViewById(R.id.profile_button);
homeButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
switchFragment(homeFragment);
}
});
productsButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
switchFragment(productsFragment);
}
});
cartButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
switchFragment(cartFragment);
}
});
profileButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
switchFragment(profileFragment);
}
});
getSupportFragmentManager().beginTransaction().add(R.id.fragment_container, homeFragment).commit();
}
private void switchFragment(Fragment fragment) {
if (activeFragment != fragment) {
getSupportFragmentManager().beginTransaction().hide(activeFragment).show(fragment).commit();
activeFragment = fragment;
}
}
}
```
其中,HomeFragment、ProductsFragment、CartFragment和ProfileFragment是你自己创建的Fragment类,用来显示对应的页面内容。在Activity中,我们使用了一个变量activeFragment来记录当前显示的Fragment,点击底部导航栏的按钮后,我们调用switchFragment()方法来切换Fragment。这个方法会先判断目标Fragment是否已经被添加到Activity中,如果没有,则添加;如果已经添加,则隐藏当前的Fragment并显示目标Fragment。
阅读全文
相关推荐
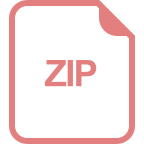
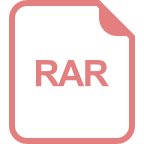

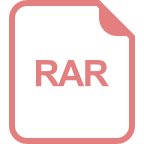
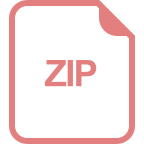
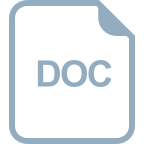

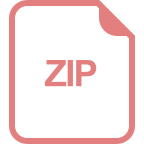
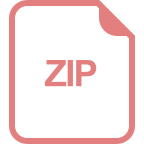
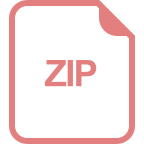
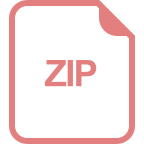
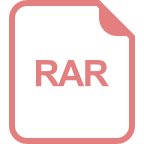
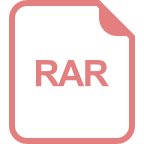
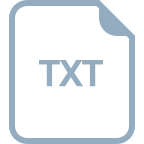
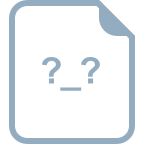
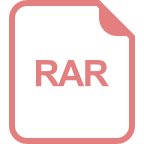
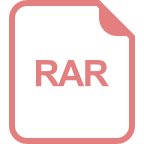
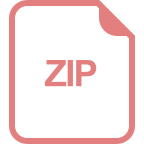