对上述代码进行不断的完善,增加对图像进行二值化处理,对图像进行腐蚀和膨胀操作,以去除噪声,查找轮廓,遍历轮廓,找到最大的矩形,找到最大矩形的四个角点,用绿色线框出车牌区域,在屏幕上显示图片和识别结果等多种详细的效果
时间: 2024-05-07 08:20:17 浏览: 16
好的,这是一个比较复杂的任务,需要使用到一些图像处理库和算法。我可以给您提供一个基本的框架,您可以根据自己的实际需求进行调整和完善。
首先,我们需要导入一些必要的库:
```python
import cv2
import numpy as np
import pytesseract
```
然后,我们读取一张车牌图片,并将其转换为灰度图像:
```python
img = cv2.imread('car_plate.jpg')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
```
接下来,我们对灰度图像进行二值化处理:
```python
ret, thresh = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY + cv2.THRESH_OTSU)
```
然后,我们进行一些形态学操作,包括腐蚀和膨胀,以去除噪声:
```python
kernel = np.ones((3,3), np.uint8)
erosion = cv2.erode(thresh, kernel, iterations=1)
dilation = cv2.dilate(erosion, kernel, iterations=1)
```
接下来,我们查找轮廓,并遍历轮廓,找到最大的矩形,以及最大矩形的四个角点:
```python
contours, hierarchy = cv2.findContours(dilation, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
max_area = 0
max_contour = None
for contour in contours:
area = cv2.contourArea(contour)
if area > max_area:
max_area = area
max_contour = contour
if max_contour is not None:
rect = cv2.minAreaRect(max_contour)
box = cv2.boxPoints(rect)
box = np.int0(box)
```
然后,我们用绿色线框出车牌区域:
```python
cv2.drawContours(img, [box], 0, (0, 255, 0), 2)
```
最后,我们使用 pytesseract 库对车牌区域进行 OCR 识别,获取识别结果,并在屏幕上显示图片和识别结果:
```python
plate_img = img[min(box[:,1]):max(box[:,1]), min(box[:,0]):max(box[:,0])]
plate_text = pytesseract.image_to_string(plate_img, lang='eng', config='--psm 11')
cv2.imshow('Plate Image', plate_img)
cv2.imshow('Result', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
print('Plate Text:', plate_text)
```
完整代码如下:
```python
import cv2
import numpy as np
import pytesseract
img = cv2.imread('car_plate.jpg')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
ret, thresh = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY + cv2.THRESH_OTSU)
kernel = np.ones((3,3), np.uint8)
erosion = cv2.erode(thresh, kernel, iterations=1)
dilation = cv2.dilate(erosion, kernel, iterations=1)
contours, hierarchy = cv2.findContours(dilation, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
max_area = 0
max_contour = None
for contour in contours:
area = cv2.contourArea(contour)
if area > max_area:
max_area = area
max_contour = contour
if max_contour is not None:
rect = cv2.minAreaRect(max_contour)
box = cv2.boxPoints(rect)
box = np.int0(box)
cv2.drawContours(img, [box], 0, (0, 255, 0), 2)
plate_img = img[min(box[:,1]):max(box[:,1]), min(box[:,0]):max(box[:,0])]
plate_text = pytesseract.image_to_string(plate_img, lang='eng', config='--psm 11')
cv2.imshow('Plate Image', plate_img)
cv2.imshow('Result', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
print('Plate Text:', plate_text)
```
请注意,该算法可能因车牌图片的质量、角度和光照等因素而产生误差。如果您需要更精确的识别结果,请考虑使用更先进的算法。
相关推荐
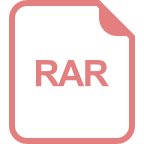
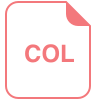
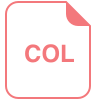











