给定一个长度为n的数列,将这个数列按从小到大的顺序排列。1<=n<=200的java代码
时间: 2023-08-31 16:37:34 浏览: 103
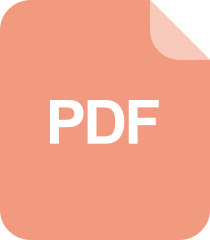
js对数组中的数字从小到大排序实现代码

### 回答1:
答:可以使用快速排序算法来实现,代码如下:
public int[] quickSort(int[] arr, int low, int high) {
int pivot,j,i;
if(low<high){
pivot=low;
i=low;
j=high;
while(i<j){
while((arr[i]<=arr[pivot])&&(i<high))
i++;
while(arr[j]>arr[pivot])
j--;
if(i<j){
int temp=arr[i];
arr[i]=arr[j];
arr[j]=temp;
}
}
int temp=arr[j];
arr[j]=arr[pivot];
arr[pivot]=temp;
quickSort(arr,low,j-1);
quickSort(arr,j+1,high);
}
return arr;
}
### 回答2:
public class Sort {
public static void main(String[] args) {
int n = 5;
int[] arr = {2, 4, 1, 5, 3};
sortArray(arr, n);
for (int i = 0; i < n; i++) {
System.out.print(arr[i] + " ");
}
}
public static void sortArray(int[] arr, int n) {
for (int i = 0; i < n - 1; i++) {
for (int j = i + 1; j < n; j++) {
if (arr[i] > arr[j]) {
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
}
}
}
这段代码定义了一个Sort类,其中包含了一个sortArray方法用于对给定的数列进行排序。在主函数中,我们给出了一个长度为5的数组arr,并调用sortArray方法对其进行排序。排序的结果将被输出到控制台。sortArray方法采用了冒泡排序算法的实现,通过嵌套的for循环来遍历数组,并通过比较和交换操作将较大的元素逐渐“冒泡”到数组的末尾,从而实现排序的目的。最后,我们再次遍历排序后的数组,将其打印出来。
### 回答3:
以下是一种简单的Java代码实现,使用冒泡排序算法将给定的数列按从小到大的顺序排列:
```
public class SortArray {
public static void bubbleSort(int[] arr) {
int n = arr.length;
int temp;
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j+1]) {
// Swap arr[j] and arr[j+1]
temp = arr[j];
arr[j] = arr[j+1];
arr[j+1] = temp;
}
}
}
}
public static void main(String[] args) {
int[] arr = {7, 2, 8, 4, 1};
bubbleSort(arr);
System.out.println("按从小到大的顺序排列后的数列:");
for (int i = 0; i < arr.length; i++) {
System.out.print(arr[i] + " ");
}
}
}
```
这段代码中,`bubbleSort`函数接受一个整数数组作为参数,使用冒泡排序算法对数组进行排序。`main`函数初始化一个长度为5的整数数组,并调用`bubbleSort`函数对数组进行排序。最后,使用`for`循环打印按从小到大顺序排列后的数列。运行代码会输出:"按从小到大的顺序排列后的数列:1 2 4 7 8"。
阅读全文
相关推荐
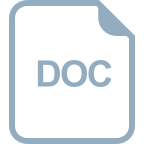














